This file contains Unicode characters that might be confused with other characters. If you think that this is intentional, you can safely ignore this warning. Use the Escape button to reveal them.
LibFile: nema_steppers.scad
Mounting holes for NEMA motors, and simple motor models.
To use, add the following lines to the beginning of your file:
include <BOSL2/std.scad>
include <BOSL2/nema_steppers.scad>
File Contents
-
nema_stepper_motor()
– Creates a NEMA standard stepper motor model. [Geom]
-
nema_mount_mask()
– Creates a standard NEMA mount holes mask. [Geom]
-
nema_motor_info()
– Returns dimension info for a given NEMA motor size.
Section: Motor Models
Module: nema_stepper_motor()
Synopsis: Creates a NEMA standard stepper motor model. [Geom]
See Also: nema_mount_mask()
Usage:
- nema_stepper_motor(size, h, shaft_len, [$slop=], ...) [ATTACHMENTS];
Description:
Creates a model of a NEMA standard stepper motor.
Arguments:
By Position | What it does |
---|---|
size |
The NEMA standard size of the stepper motor. |
h |
Length of motor body. Default: 24mm |
shaft_len |
Length of shaft protruding out the top of the stepper motor. Default: 20mm |
By Name | What it does |
---|---|
details |
If false, creates a very rough motor shape, suitable for using as a mask. Default: true |
atype |
The attachment set type to use when anchoring. Default: "body" |
$slop |
If details is false then increase size of the model by double this amount (for use as a mask) |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: TOP |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
orient |
Vector to rotate top towards, after spin. See orient. Default: UP |
Anchor Types:
Anchor Type | What it is |
---|---|
"shaft" | Anchor relative to the shaft. |
"plinth" | Anchor relative to the plinth. |
"body" | Anchor relative to the motor body. |
"screws" | Anchor relative to the screw hole centers. ie: TOP+RIGHT+FRONT is the center-top of the front-right screwhole. |
Example 1:
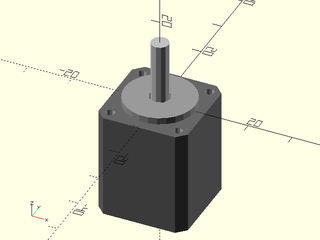
include <BOSL2/std.scad>
include <BOSL2/nema_steppers.scad>
nema_stepper_motor(size=8, h=24, shaft_len=15);
Example 2:
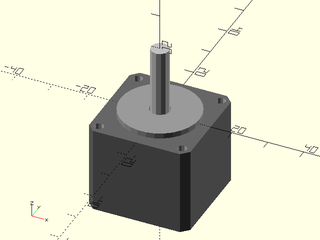
include <BOSL2/std.scad>
include <BOSL2/nema_steppers.scad>
nema_stepper_motor(size=11, h=24, shaft_len=20);
Example 3:
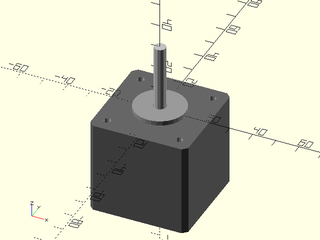
include <BOSL2/std.scad>
include <BOSL2/nema_steppers.scad>
nema_stepper_motor(size=17, h=40, shaft_len=30);
Example 4:
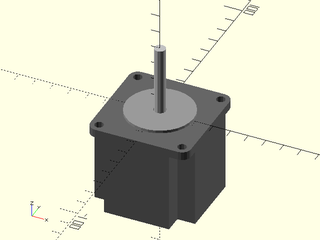
include <BOSL2/std.scad>
include <BOSL2/nema_steppers.scad>
nema_stepper_motor(size=23, h=50, shaft_len=40);
Example 5:
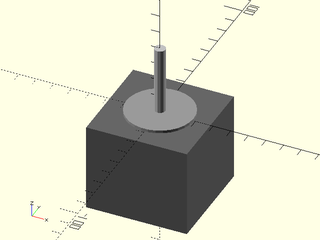
include <BOSL2/std.scad>
include <BOSL2/nema_steppers.scad>
nema_stepper_motor(size=23, h=50, shaft_len=40, details=false);
Section: Masking Modules
Module: nema_mount_mask()
Synopsis: Creates a standard NEMA mount holes mask. [Geom]
See Also: nema_stepper_motor()
Usage:
- nema_mount_mask(size, depth, l, [$slop], ...);
Description:
Creates a mask to use when making standard NEMA stepper motor mounts.
Arguments:
By Position | What it does |
---|---|
size |
The standard NEMA motor size to make a mount for. |
depth |
The thickness of the mounting hole mask. Default: 5 |
l |
The length of the slots, for making an adjustable motor mount. Default: 5 |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
orient |
Vector to rotate top towards, after spin. See orient. Default: UP |
$slop |
The printer-specific slop value to make parts fit just right. |
Anchor Types:
Anchor Type | What it is |
---|---|
"full" | Anchor relative the full mask. |
"screws" | Anchor relative to the screw hole centers. ie: TOP+RIGHT+FRONT is the center-top of the front-right screwhole. |
Example 1:
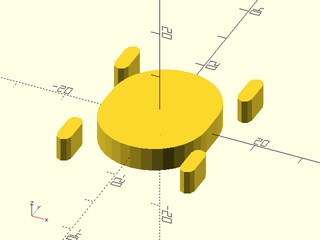
include <BOSL2/std.scad>
include <BOSL2/nema_steppers.scad>
nema_mount_mask(size=14, depth=5, l=5);
Example 2:
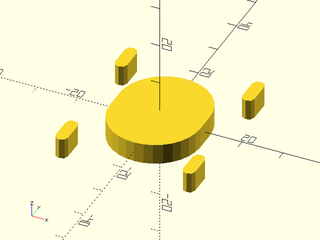
include <BOSL2/std.scad>
include <BOSL2/nema_steppers.scad>
nema_mount_mask(size=17, depth=5, l=5);
Example 3:
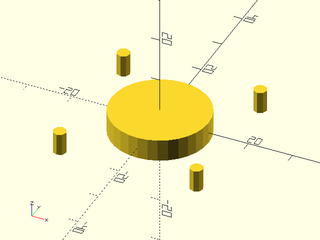
include <BOSL2/std.scad>
include <BOSL2/nema_steppers.scad>
nema_mount_mask(size=17, depth=5, l=0);
Section: Functions
Function: nema_motor_info()
Synopsis: Returns dimension info for a given NEMA motor size.
See Also: nema_stepper_motor(), nema_mount_mask()
Usage:
- info = nema_motor_info(size);
Description:
Gets various dimension info for a NEMA stepper motor of a specific size. Returns a list of scalar values, containing, in order:
- MOTOR_WIDTH: The full width and length of the motor.
- PLINTH_HEIGHT: The height of the circular plinth on the face of the motor.
- PLINTH_DIAM: The diameter of the circular plinth on the face of the motor.
- SCREW_SPACING: The spacing between screwhole centers in both X and Y axes.
- SCREW_SIZE: The diameter of the screws.
- SCREW_DEPTH: The depth of the screwholes.
- SHAFT_DIAM: The diameter of the motor shaft.
Arguments:
By Position | What it does |
---|---|
size |
The standard NEMA motor size. |
Indices
Table of Contents
Function Index
Topics Index
Cheat Sheet
Tutorials
List of Files:
Basic Modeling:
- constants.scad STD
- transforms.scad STD
- attachments.scad STD
- shapes2d.scad STD
- shapes3d.scad STD
- drawing.scad STD
- masks2d.scad STD
- masks3d.scad STD
- distributors.scad STD
- color.scad STD
- partitions.scad STD
- miscellaneous.scad STD
Advanced Modeling:
- paths.scad STD
- regions.scad STD
- skin.scad STD
- vnf.scad STD
- beziers.scad
- nurbs.scad
- rounding.scad
- turtle3d.scad
Math:
- math.scad STD
- linalg.scad STD
- vectors.scad STD
- coords.scad STD
- geometry.scad STD
- trigonometry.scad STD
Data Management:
- version.scad STD
- comparisons.scad STD
- lists.scad STD
- utility.scad STD
- strings.scad STD
- structs.scad STD
- fnliterals.scad
Threaded Parts:
Parts:
- ball_bearings.scad
- cubetruss.scad
- gears.scad
- hinges.scad
- joiners.scad
- linear_bearings.scad
- modular_hose.scad
- nema_steppers.scad
- polyhedra.scad
- sliders.scad
- tripod_mounts.scad
- walls.scad
- wiring.scad
Footnotes:
STD = Included in std.scad