Table of Contents
- LibFile: shapes2d.scad
- File Contents
- Section: 2D Primitives
- Function/Module: square()
- Function/Module: rect()
- Function/Module: circle()
- Function/Module: ellipse()
- Section: Polygons
- Function/Module: regular_ngon()
- Function/Module: pentagon()
- Function/Module: hexagon()
- Function/Module: octagon()
- Function/Module: right_triangle()
- Function/Module: trapezoid()
- Function/Module: star()
- Module: jittered_poly()
- Section: Curved 2D Shapes
- Function/Module: teardrop2d()
- Function/Module: egg()
- Function/Module: ring()
- Function/Module: glued_circles()
- Function/Module: squircle()
- Function/Module: keyhole()
- Function/Module: supershape()
- Function/Module: reuleaux_polygon()
- Section: Text
- Section: Rounding 2D shapes
This file contains Unicode characters that might be confused with other characters. If you think that this is intentional, you can safely ignore this warning. Use the Escape button to reveal them.
LibFile: shapes2d.scad
This file includes redefinitions of the core modules to work with attachment, and functional forms of those modules that produce paths. You can create regular polygons with optional rounded corners and alignment features not available with circle(). The file also provides teardrop2d, which is useful for 3D printable holes. Many of the commands have module forms that produce geometry and function forms that produce a path.
To use, add the following lines to the beginning of your file:
include <BOSL2/std.scad>
File Contents
-
regular_ngon()
– Creates a regular N-sided polygon. [Geom] [Path]pentagon()
– Creates a regular pentagon. [Geom] [Path]hexagon()
– Creates a regular hexagon. [Geom] [Path]octagon()
– Creates a regular octagon. [Geom] [Path]right_triangle()
– Creates a right triangle. [Geom] [Path]trapezoid()
– Creates a trapezoid with parallel top and bottom sides. [Geom] [Path]star()
– Creates a star-shaped polygon or returns a star-shaped region. [Geom] [Path]jittered_poly()
– Creates a polygon with extra points for smoother twisted extrusions. [Geom]
-
teardrop2d()
– Creates a 2D teardrop shape. [Geom] [Path]egg()
– Creates an egg-shaped 2d object. [Geom] [Path]ring()
– Draws a 2D ring or partial ring or returns a region or path [Geom] [Region] [Path]glued_circles()
– Creates a shape of two circles joined by a curved waist. [Geom] [Path]squircle()
– Creates a shape between a circle and a square, centered on the origin. [Geom] [Path]keyhole()
– Creates a 2D keyhole shape. [Geom] [Path]supershape()
– Creates a 2D Superformula shape. [Geom] [Path]reuleaux_polygon()
– Creates a constant-width shape that is not circular. [Geom] [Path]
-
text()
– Creates an attachable block of text. [Geom]
Section: 2D Primitives
Function/Module: square()
Synopsis: Creates a 2D square or rectangle. [Geom] [Path] [Ext]
Topics: Shapes (2D), Path Generators (2D)
See Also: rect()
Usage: As a Module
- square(size, [center], ...);
Usage: With Attachments
- square(size, [center], ...) [ATTACHMENTS];
Usage: As a Function
- path = square(size, [center], ...);
Description:
When called as the built-in module, creates a 2D square or rectangle of the given size. When called as a function, returns a 2D path/list of points for a square/rectangle of the given size.
Arguments:
By Position | What it does |
---|---|
size |
The size of the square to create. If given as a scalar, both X and Y will be the same size. |
center |
If given and true, overrides anchor to be CENTER . If given and false, overrides anchor to be FRONT+LEFT . |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Example 1:
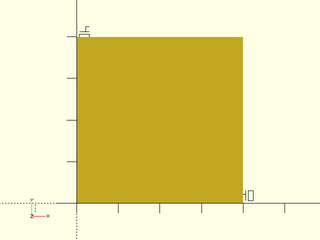
include <BOSL2/std.scad>
square(40);
Example 2: Centered
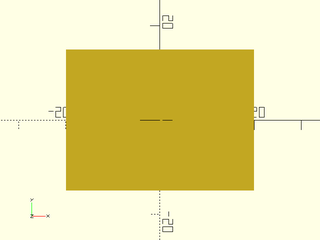
include <BOSL2/std.scad>
square([40,30], center=true);
Example 3: Called as Function
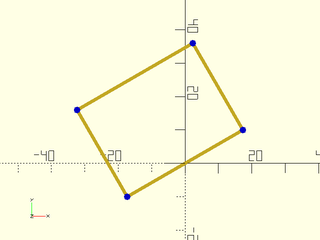
include <BOSL2/std.scad>
path = square([40,30], anchor=FRONT, spin=30);
stroke(path, closed=true);
move_copies(path) color("blue") circle(d=2,$fn=8);
Function/Module: rect()
Synopsis: Creates a 2d rectangle with optional corner rounding. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: square()
Usage: As Module
- rect(size, [rounding], [chamfer], ...) [ATTACHMENTS];
Usage: As Function
- path = rect(size, [rounding], [chamfer], ...);
Description:
When called as a module, creates a 2D rectangle of the given size, with optional rounding or chamfering. When called as a function, returns a 2D path/list of points for a square/rectangle of the given size.
Arguments:
By Position | What it does |
---|---|
size |
The size of the rectangle to create. If given as a scalar, both X and Y will be the same size. |
By Name | What it does |
---|---|
rounding |
The rounding radius for the corners. If negative, produces external roundover spikes on the X axis. If given as a list of four numbers, gives individual radii for each corner, in the order [X+Y+,X-Y+,X-Y-,X+Y-]. Default: 0 (no rounding) |
chamfer |
The chamfer size for the corners. If negative, produces external chamfer spikes on the X axis. If given as a list of four numbers, gives individual chamfers for each corner, in the order [X+Y+,X-Y+,X-Y-,X+Y-]. Default: 0 (no chamfer) |
corner_flip |
Flips the direction of the rouding curve or roudover and chamfer spikes. If true it produces spikes on the Y axis. If false it produces spikes on the X axis. If given as a list of four booleans it flips the direction for each corner, in the order [X+Y+,X-Y+,X-Y-,X+Y-]. Default: false (no flip) |
atype |
The type of anchoring to use with anchor= . Valid opptions are "box" and "perim". This lets you choose between putting anchors on the rounded or chamfered perimeter, or on the square bounding box of the shape. Default: "box" |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Anchor Types:
Anchor Type | What it is |
---|---|
box | Anchor is with respect to the rectangular bounding box of the shape. |
perim | Anchors are placed along the rounded or chamfered perimeter of the shape. |
Example 1:
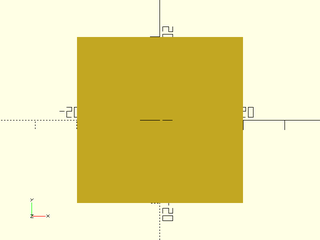
include <BOSL2/std.scad>
rect(40);
Example 2: Anchored
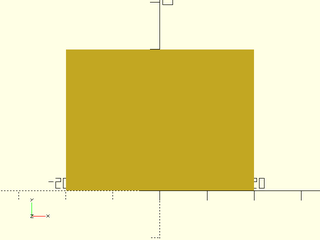
include <BOSL2/std.scad>
rect([40,30], anchor=FRONT);
Example 3: Spun
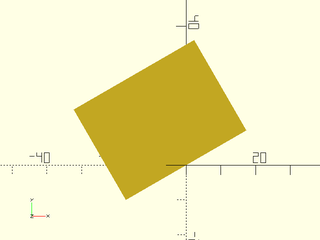
include <BOSL2/std.scad>
rect([40,30], anchor=FRONT, spin=30);
Example 4: Chamferred Rect
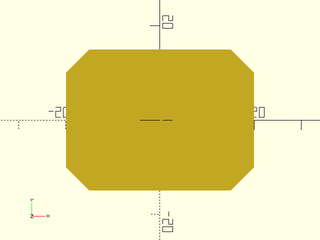
include <BOSL2/std.scad>
rect([40,30], chamfer=5);
Example 5: Rounded Rect
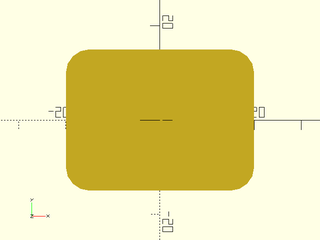
include <BOSL2/std.scad>
rect([40,30], rounding=5);
Example 6: Negative-Chamferred Rect
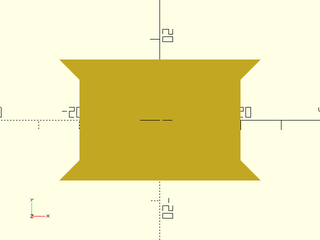
include <BOSL2/std.scad>
rect([40,30], chamfer=-5);
Example 7: Negative-Rounded Rect
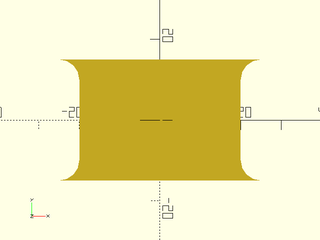
include <BOSL2/std.scad>
rect([40,30], rounding=-5);
Example 8: Combined Rounded-Chamfered Rect with corner flips
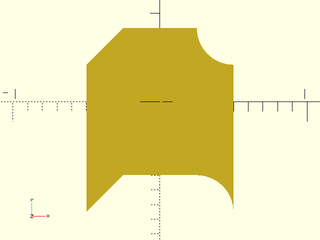
include <BOSL2/std.scad>
rect([1,1], chamfer = 0.25*[0,1,-1,0],
rounding=.25*[1,0,0,-1], corner_flip = true, $fn=32);
Example 9: Default "box" Anchors
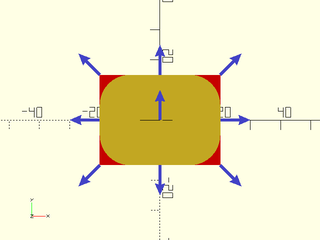
include <BOSL2/std.scad>
color("red") rect([40,30]);
rect([40,30], rounding=10)
show_anchors();
Example 10: "perim" Anchors
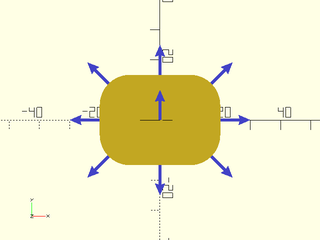
include <BOSL2/std.scad>
rect([40,30], rounding=10, atype="perim")
show_anchors();
Example 11: "perim" Anchors
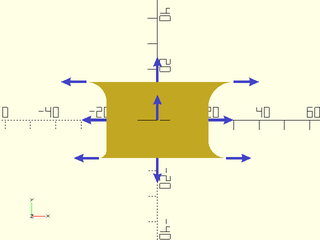
include <BOSL2/std.scad>
rect([40,30], rounding=[-10,-8,-3,-7], atype="perim")
show_anchors();
Example 12: Mixed Chamferring and Rounding
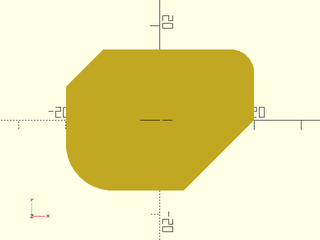
include <BOSL2/std.scad>
rect([40,30],rounding=[5,0,10,0],chamfer=[0,8,0,15],$fa=1,$fs=1);
Example 13: Called as Function
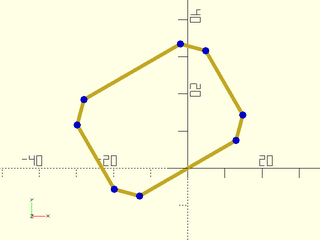
include <BOSL2/std.scad>
path = rect([40,30], chamfer=5, anchor=FRONT, spin=30);
stroke(path, closed=true);
move_copies(path) color("blue") circle(d=2,$fn=8);
Function/Module: circle()
Synopsis: Creates the approximation of a circle. [Geom] [Path] [Ext]
Topics: Shapes (2D), Path Generators (2D)
See Also: ellipse(), circle_2tangents(), circle_3points()
Usage: As a Module
- circle(r|d=, ...) [ATTACHMENTS];
- circle(points=) [ATTACHMENTS];
- circle(r|d=, corner=) [ATTACHMENTS];
Usage: As a Function
- path = circle(r|d=, ...);
- path = circle(points=);
- path = circle(r|d=, corner=);
Description:
When called as the built-in module, creates a 2D polygon that approximates a circle of the given size.
When called as a function, returns a 2D list of points (path) for a polygon that approximates a circle of the given size.
If corner=
is given three 2D points, centers the circle so that it will be tangent to both segments of the path, on the inside corner.
If points=
is given three 2D points, centers and sizes the circle so that it passes through all three points.
Arguments:
By Position | What it does |
---|---|
r |
The radius of the circle to create. |
d |
The diameter of the circle to create. |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Example 1: By Radius
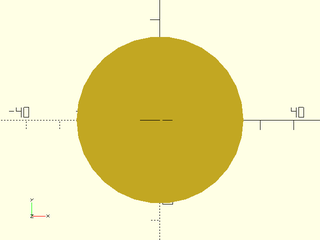
include <BOSL2/std.scad>
circle(r=25);
Example 2: By Diameter
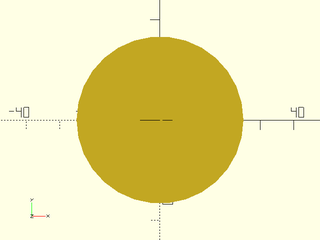
include <BOSL2/std.scad>
circle(d=50);
Example 3: Fit to Three Points
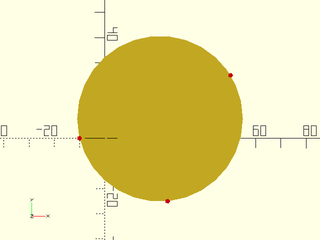
include <BOSL2/std.scad>
pts = [[50,25], [25,-25], [-10,0]];
circle(points=pts);
color("red") move_copies(pts) circle();
Example 4: Fit Tangent to Inside Corner of Two Segments
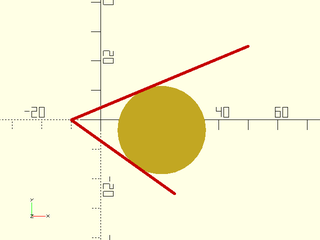
include <BOSL2/std.scad>
path = [[50,25], [-10,0], [25,-25]];
circle(corner=path, r=15);
color("red") stroke(path);
Example 5: Called as Function
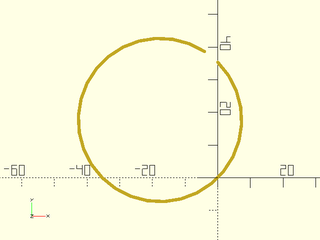
include <BOSL2/std.scad>
path = circle(d=50, anchor=FRONT, spin=45);
stroke(path);
Function/Module: ellipse()
Synopsis: Creates the approximation of an ellipse or a circle. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: circle(), circle_2tangents(), circle_3points()
Usage: As a Module
- ellipse(r|d=, [realign=], [circum=], [uniform=], ...) [ATTACHMENTS];
Usage: As a Function
- path = ellipse(r|d=, [realign=], [circum=], [uniform=], ...);
Description:
When called as a module, creates a 2D polygon that approximates a circle or ellipse of the given size.
When called as a function, returns a 2D list of points (path) for a polygon that approximates a circle or ellipse of the given size.
By default the point list or shape is the same as the one you would get by scaling the output of circle()
, but with this module your
attachments to the ellipse will retain their dimensions, whereas scaling a circle with attachments will also scale the attachments.
If you set uniform
to true then you will get a polygon with congruent sides whose vertices lie on the ellipse. The circum
option
requests a polygon that circumscribes the requested ellipse (so the specified ellipse will fit into the resulting polygon). Note that
you cannot gives circum=true
and uniform=true
.
Arguments:
By Position | What it does |
---|---|
r |
Radius of the circle or pair of semiaxes of ellipse |
By Name | What it does |
---|---|
d |
Diameter of the circle or a pair giving the full X and Y axis lengths. |
realign |
If false starts the approximate ellipse with a point on the X+ axis. If true the midpoint of a side is on the X+ axis and the first point of the polygon is below the X+ axis. This can result in a very different polygon when $fn is small. Default: false |
uniform |
If true, the polygon that approximates the circle will have segments of equal length. Only works if circum=false . Default: false |
circum |
If true, the polygon that approximates the circle will be upsized slightly to circumscribe the theoretical circle. If false, it inscribes the theoretical circle. If this is true then uniform must be false. Default: false |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Example 1: By Radius
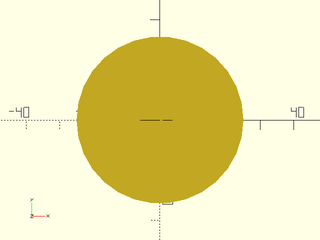
include <BOSL2/std.scad>
ellipse(r=25);
Example 2: By Diameter
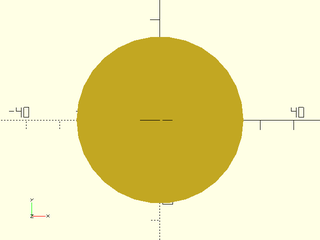
include <BOSL2/std.scad>
ellipse(d=50);
Example 3: Anchoring
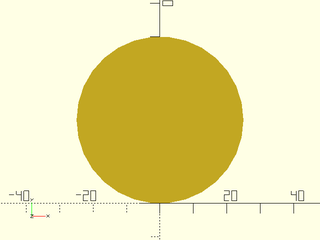
include <BOSL2/std.scad>
ellipse(d=50, anchor=FRONT);
Example 4: Spin
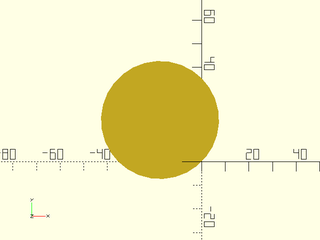
include <BOSL2/std.scad>
ellipse(d=50, anchor=FRONT, spin=45);
Example 5: Called as Function
include <BOSL2/std.scad>
path = ellipse(d=50, anchor=FRONT, spin=45);
Example 6: Uniformly sampled hexagon at the top, regular non-uniform one at the bottom
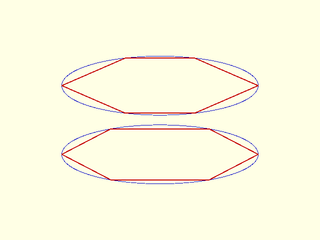
include <BOSL2/std.scad>
r=[10,3];
ydistribute(7){
union(){
stroke([ellipse(r=r, $fn=100)],width=0.05,color="blue");
stroke([ellipse(r=r, $fn=6)],width=0.1,color="red");
}
union(){
stroke([ellipse(r=r, $fn=100)],width=0.05,color="blue");
stroke([ellipse(r=r, $fn=6,uniform=true)],width=0.1,color="red");
}
}
Example 7: The realigned hexagons are even more different
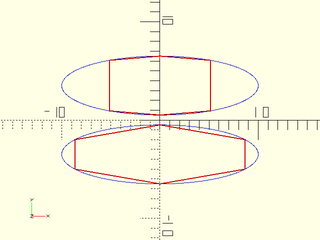
include <BOSL2/std.scad>
r=[10,3];
ydistribute(7){
union(){
stroke([ellipse(r=r, $fn=100)],width=0.05,color="blue");
stroke([ellipse(r=r, $fn=6,realign=true)],width=0.1,color="red");
}
union(){
stroke([ellipse(r=r, $fn=100)],width=0.05,color="blue");
stroke([ellipse(r=r, $fn=6,realign=true,uniform=true)],width=0.1,color="red");
}
}
Example 8: For odd $fn the result may not look very elliptical:
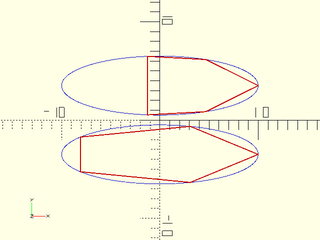
include <BOSL2/std.scad>
r=[10,3];
ydistribute(7){
union(){
stroke([ellipse(r=r, $fn=100)],width=0.05,color="blue");
stroke([ellipse(r=r, $fn=5,realign=false)],width=0.1,color="red");
}
union(){
stroke([ellipse(r=r, $fn=100)],width=0.05,color="blue");
stroke([ellipse(r=r, $fn=5,realign=false,uniform=true)],width=0.1,color="red");
}
}
Example 9: The same ellipse, turned 90 deg, gives a very different result:
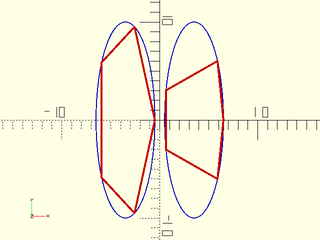
include <BOSL2/std.scad>
r=[3,10];
xdistribute(7){
union(){
stroke([ellipse(r=r, $fn=100)],width=0.1,color="blue");
stroke([ellipse(r=r, $fn=5,realign=false)],width=0.2,color="red");
}
union(){
stroke([ellipse(r=r, $fn=100)],width=0.1,color="blue");
stroke([ellipse(r=r, $fn=5,realign=false,uniform=true)],width=0.2,color="red");
}
}
Section: Polygons
Function/Module: regular_ngon()
Synopsis: Creates a regular N-sided polygon. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: debug_polygon(), circle(), pentagon(), hexagon(), octagon(), ellipse(), star()
Usage:
- regular_ngon(n, r|d=|or=|od=, [realign=]) [ATTACHMENTS];
- regular_ngon(n, ir=|id=, [realign=]) [ATTACHMENTS];
- regular_ngon(n, side=, [realign=]) [ATTACHMENTS];
Description:
When called as a function, returns a 2D path for a regular N-sided polygon. When called as a module, creates a 2D regular N-sided polygon.
Arguments:
By Position | What it does |
---|---|
n |
The number of sides. |
r / or |
Outside radius, at points. |
By Name | What it does |
---|---|
d / od |
Outside diameter, at points. |
ir |
Inside radius, at center of sides. |
id |
Inside diameter, at center of sides. |
side |
Length of each side. |
rounding |
Radius of rounding for the tips of the polygon. Default: 0 (no rounding) |
realign |
If false, vertex 0 will lie on the X+ axis. If true then the midpoint of the last edge will lie on the X+ axis, and vertex 0 will be below the X axis. Default: false |
align_tip |
If given as a 2D vector, rotates the whole shape so that the first vertex points in that direction. This occurs before spin. |
align_side |
If given as a 2D vector, rotates the whole shape so that the normal of side0 points in that direction. This occurs before spin. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Named Anchors:
Anchor Name | Position |
---|---|
"tip0", "tip1", etc. | Each tip has an anchor, pointing outwards. |
"side0", "side1", etc. | The center of each side has an anchor, pointing outwards. |
Example 1: by Outer Size
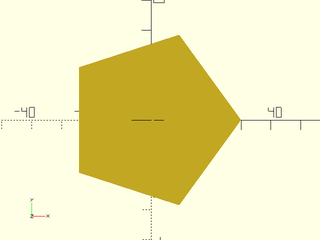
include <BOSL2/std.scad>
regular_ngon(n=5, or=30);
regular_ngon(n=5, od=60);
Example 2: by Inner Size
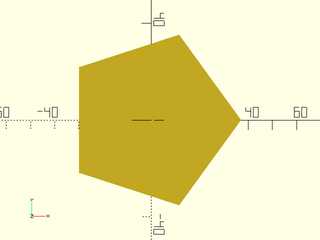
include <BOSL2/std.scad>
regular_ngon(n=5, ir=30);
regular_ngon(n=5, id=60);
Example 3: by Side Length
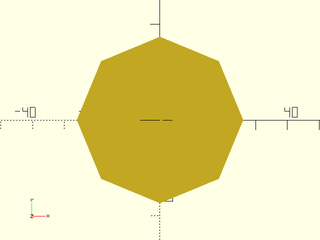
include <BOSL2/std.scad>
regular_ngon(n=8, side=20);
Example 4: Realigned
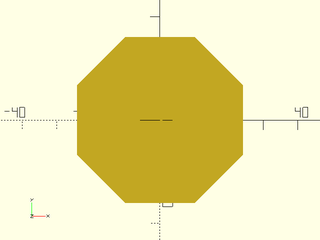
include <BOSL2/std.scad>
regular_ngon(n=8, side=20, realign=true);
Example 5: Alignment by Tip
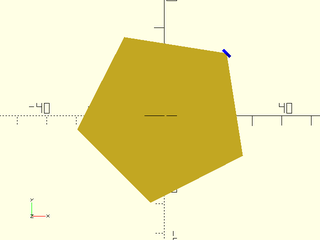
include <BOSL2/std.scad>
regular_ngon(n=5, r=30, align_tip=BACK+RIGHT)
attach("tip0", FWD) color("blue")
stroke([[0,0],[0,7]], endcap2="arrow2");
Example 6: Alignment by Side
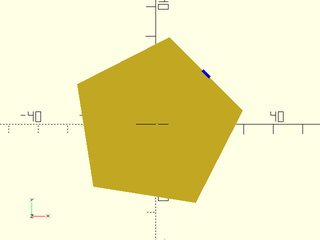
include <BOSL2/std.scad>
regular_ngon(n=5, r=30, align_side=BACK+RIGHT)
attach("side0", FWD) color("blue")
stroke([[0,0],[0,7]], endcap2="arrow2");
Example 7: Rounded
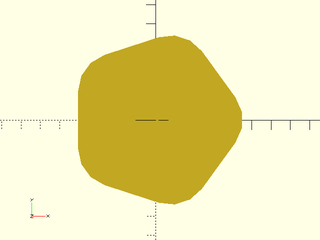
include <BOSL2/std.scad>
regular_ngon(n=5, od=100, rounding=20, $fn=20);
Example 8: Called as Function
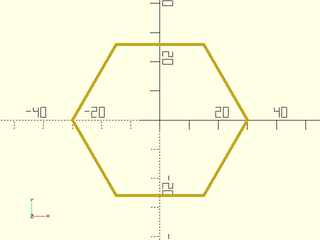
include <BOSL2/std.scad>
stroke(closed=true, regular_ngon(n=6, or=30));
Function/Module: pentagon()
Synopsis: Creates a regular pentagon. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: circle(), regular_ngon(), hexagon(), octagon(), ellipse(), star()
Usage:
- pentagon(or|od=, [realign=], [align_tip=|align_side=]) [ATTACHMENTS];
- pentagon(ir=|id=, [realign=], [align_tip=|align_side=]) [ATTACHMENTS];
- pentagon(side=, [realign=], [align_tip=|align_side=]) [ATTACHMENTS];
Usage: as function
- path = pentagon(...);
Description:
When called as a function, returns a 2D path for a regular pentagon. When called as a module, creates a 2D regular pentagon.
Arguments:
By Position | What it does |
---|---|
r / or |
Outside radius, at points. |
By Name | What it does |
---|---|
d / od |
Outside diameter, at points. |
ir |
Inside radius, at center of sides. |
id |
Inside diameter, at center of sides. |
side |
Length of each side. |
rounding |
Radius of rounding for the tips of the polygon. Default: 0 (no rounding) |
realign |
If false, vertex 0 will lie on the X+ axis. If true then the midpoint of the last edge will lie on the X+ axis, and vertex 0 will be below the X axis. Default: false |
align_tip |
If given as a 2D vector, rotates the whole shape so that the first vertex points in that direction. This occurs before spin. |
align_side |
If given as a 2D vector, rotates the whole shape so that the normal of side0 points in that direction. This occurs before spin. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Named Anchors:
Anchor Name | Position |
---|---|
"tip0" ... "tip4" | Each tip has an anchor, pointing outwards. |
"side0" ... "side4" | The center of each side has an anchor, pointing outwards. |
Example 1: by Outer Size
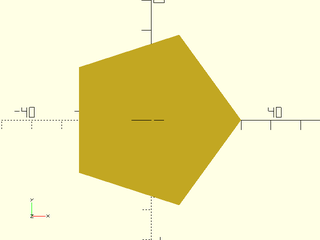
include <BOSL2/std.scad>
pentagon(or=30);
pentagon(od=60);
Example 2: by Inner Size
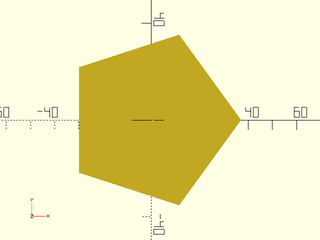
include <BOSL2/std.scad>
pentagon(ir=30);
pentagon(id=60);
Example 3: by Side Length
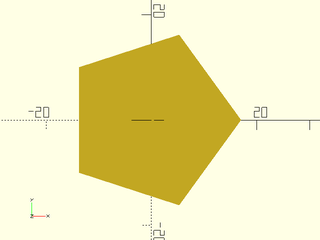
include <BOSL2/std.scad>
pentagon(side=20);
Example 4: Realigned
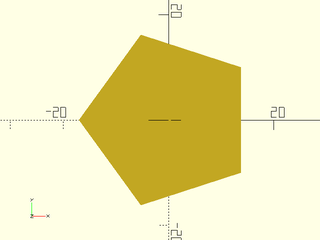
include <BOSL2/std.scad>
pentagon(side=20, realign=true);
Example 5: Alignment by Tip
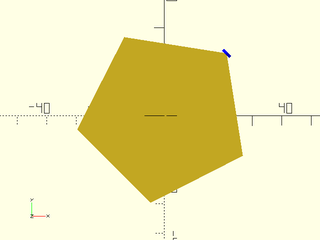
include <BOSL2/std.scad>
pentagon(r=30, align_tip=BACK+RIGHT)
attach("tip0", FWD) color("blue")
stroke([[0,0],[0,7]], endcap2="arrow2");
Example 6: Alignment by Side
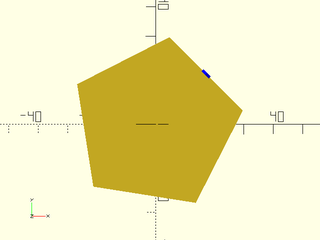
include <BOSL2/std.scad>
pentagon(r=30, align_side=BACK+RIGHT)
attach("side0", FWD) color("blue")
stroke([[0,0],[0,7]], endcap2="arrow2");
Example 7: Rounded
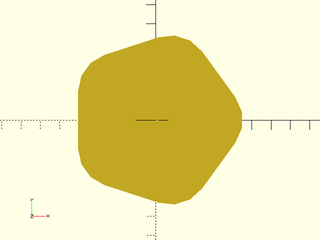
include <BOSL2/std.scad>
pentagon(od=100, rounding=20, $fn=20);
Example 8: Called as Function
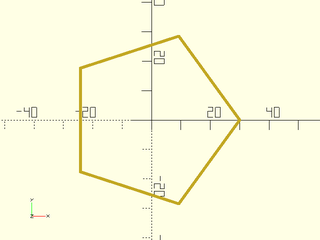
include <BOSL2/std.scad>
stroke(closed=true, pentagon(or=30));
Function/Module: hexagon()
Synopsis: Creates a regular hexagon. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: circle(), regular_ngon(), pentagon(), octagon(), ellipse(), star()
Usage: As Module
- hexagon(r/or, [realign=], <align_tip=|align_side=>, [rounding=], ...) [ATTACHMENTS];
- hexagon(d=/od=, ...) [ATTACHMENTS];
- hexagon(ir=/id=, ...) [ATTACHMENTS];
- hexagon(side=, ...) [ATTACHMENTS];
Usage: As Function
- path = hexagon(...);
Description:
When called as a function, returns a 2D path for a regular hexagon. When called as a module, creates a 2D regular hexagon.
Arguments:
By Position | What it does |
---|---|
r / or |
Outside radius, at points. |
By Name | What it does |
---|---|
d / od |
Outside diameter, at points. |
ir |
Inside radius, at center of sides. |
id |
Inside diameter, at center of sides. |
side |
Length of each side. |
rounding |
Radius of rounding for the tips of the polygon. Default: 0 (no rounding) |
realign |
If false, vertex 0 will lie on the X+ axis. If true then the midpoint of the last edge will lie on the X+ axis, and vertex 0 will be below the X axis. Default: false |
align_tip |
If given as a 2D vector, rotates the whole shape so that the first vertex points in that direction. This occurs before spin. |
align_side |
If given as a 2D vector, rotates the whole shape so that the normal of side0 points in that direction. This occurs before spin. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Named Anchors:
Anchor Name | Position |
---|---|
"tip0" ... "tip5" | Each tip has an anchor, pointing outwards. |
"side0" ... "side5" | The center of each side has an anchor, pointing outwards. |
Example 1: by Outer Size
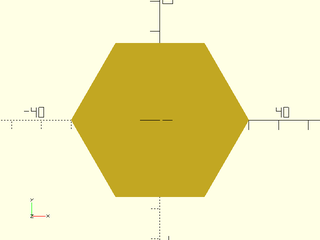
include <BOSL2/std.scad>
hexagon(or=30);
hexagon(od=60);
Example 2: by Inner Size
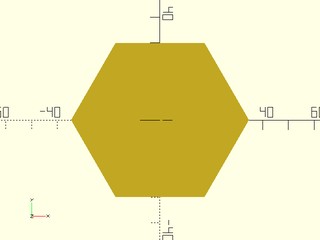
include <BOSL2/std.scad>
hexagon(ir=30);
hexagon(id=60);
Example 3: by Side Length
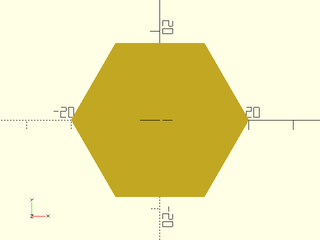
include <BOSL2/std.scad>
hexagon(side=20);
Example 4: Realigned
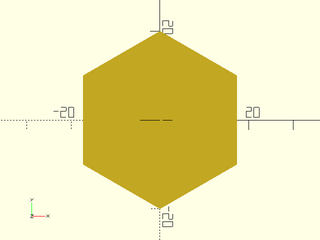
include <BOSL2/std.scad>
hexagon(side=20, realign=true);
Example 5: Alignment by Tip
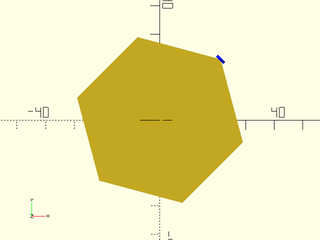
include <BOSL2/std.scad>
hexagon(r=30, align_tip=BACK+RIGHT)
attach("tip0", FWD) color("blue")
stroke([[0,0],[0,7]], endcap2="arrow2");
Example 6: Alignment by Side
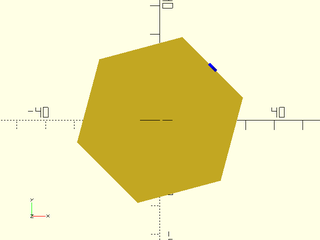
include <BOSL2/std.scad>
hexagon(r=30, align_side=BACK+RIGHT)
attach("side0", FWD) color("blue")
stroke([[0,0],[0,7]], endcap2="arrow2");
Example 7: Rounded
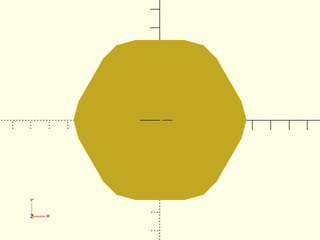
include <BOSL2/std.scad>
hexagon(od=100, rounding=20, $fn=20);
Example 8: Called as Function
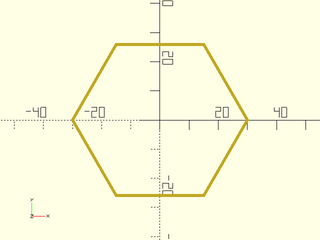
include <BOSL2/std.scad>
stroke(closed=true, hexagon(or=30));
Function/Module: octagon()
Synopsis: Creates a regular octagon. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: circle(), regular_ngon(), pentagon(), hexagon(), ellipse(), star()
Usage: As Module
- octagon(r/or, [realign=], [align_tip=|align_side=], [rounding=], ...) [ATTACHMENTS];
- octagon(d=/od=, ...) [ATTACHMENTS];
- octagon(ir=/id=, ...) [ATTACHMENTS];
- octagon(side=, ...) [ATTACHMENTS];
Usage: As Function
- path = octagon(...);
Description:
When called as a function, returns a 2D path for a regular octagon. When called as a module, creates a 2D regular octagon.
Arguments:
By Position | What it does |
---|---|
r / or |
Outside radius, at points. |
d / od |
Outside diameter, at points. |
ir |
Inside radius, at center of sides. |
id |
Inside diameter, at center of sides. |
side |
Length of each side. |
rounding |
Radius of rounding for the tips of the polygon. Default: 0 (no rounding) |
realign |
If false, vertex 0 will lie on the X+ axis. If true then the midpoint of the last edge will lie on the X+ axis, and vertex 0 will be below the X axis. Default: false |
align_tip |
If given as a 2D vector, rotates the whole shape so that the first vertex points in that direction. This occurs before spin. |
align_side |
If given as a 2D vector, rotates the whole shape so that the normal of side0 points in that direction. This occurs before spin. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Named Anchors:
Anchor Name | Position |
---|---|
"tip0" ... "tip7" | Each tip has an anchor, pointing outwards. |
"side0" ... "side7" | The center of each side has an anchor, pointing outwards. |
Example 1: by Outer Size
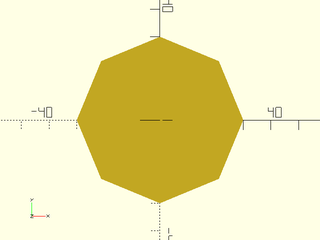
include <BOSL2/std.scad>
octagon(or=30);
octagon(od=60);
Example 2: by Inner Size
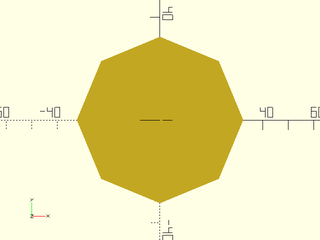
include <BOSL2/std.scad>
octagon(ir=30);
octagon(id=60);
Example 3: by Side Length
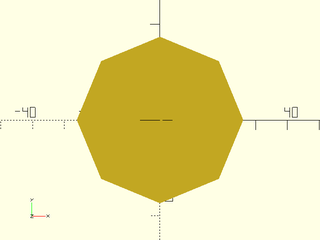
include <BOSL2/std.scad>
octagon(side=20);
Example 4: Realigned
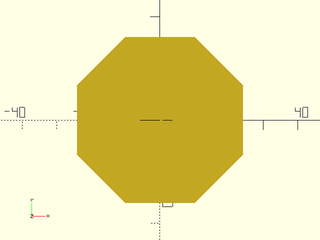
include <BOSL2/std.scad>
octagon(side=20, realign=true);
Example 5: Alignment by Tip
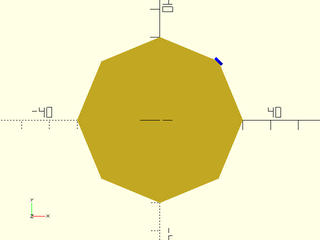
include <BOSL2/std.scad>
octagon(r=30, align_tip=BACK+RIGHT)
attach("tip0", FWD) color("blue")
stroke([[0,0],[0,7]], endcap2="arrow2");
Example 6: Alignment by Side
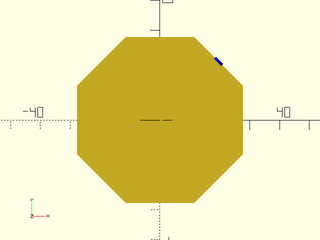
include <BOSL2/std.scad>
octagon(r=30, align_side=BACK+RIGHT)
attach("side0", FWD) color("blue")
stroke([[0,0],[0,7]], endcap2="arrow2");
Example 7: Rounded
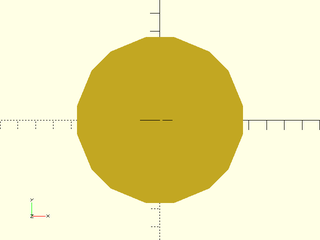
include <BOSL2/std.scad>
octagon(od=100, rounding=20, $fn=20);
Example 8: Called as Function
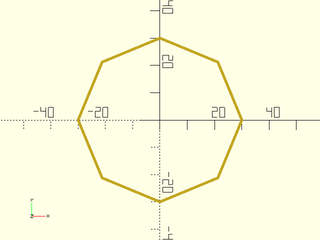
include <BOSL2/std.scad>
stroke(closed=true, octagon(or=30));
Function/Module: right_triangle()
Synopsis: Creates a right triangle. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: square(), rect(), regular_ngon(), pentagon(), hexagon(), octagon(), star()
Usage: As Module
- right_triangle(size, [center], ...) [ATTACHMENTS];
Usage: As Function
- path = right_triangle(size, [center], ...);
Description:
When called as a module, creates a right triangle with the Hypotenuse in the X+Y+ quadrant. When called as a function, returns a 2D path for a right triangle with the Hypotenuse in the X+Y+ quadrant.
Arguments:
By Position | What it does |
---|---|
size |
The width and length of the right triangle, given as a scalar or an XY vector. |
center |
If true, forces anchor=CENTER . If false, forces anchor=[-1,-1] . Default: undef (use anchor= ) |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Named Anchors:
Anchor Name | Position |
---|---|
"hypot" | Center of angled side, perpendicular to that side. |
Example 1:
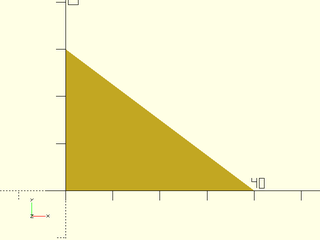
include <BOSL2/std.scad>
right_triangle([40,30]);
Example 2: With center=true
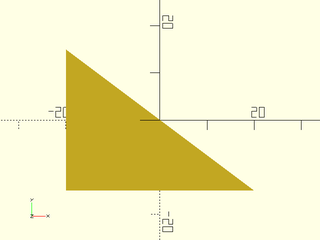
include <BOSL2/std.scad>
right_triangle([40,30], center=true);
Example 3: Standard Anchors
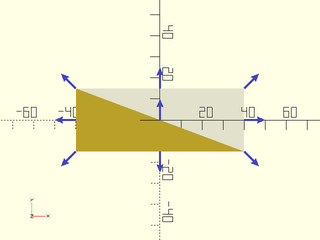
include <BOSL2/std.scad>
right_triangle([80,30], center=true)
show_anchors(custom=false);
color([0.5,0.5,0.5,0.1])
square([80,30], center=true);
Example 4: Named Anchors
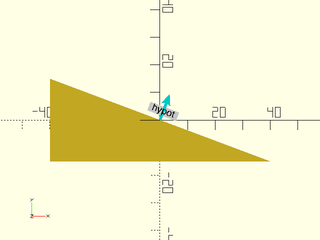
include <BOSL2/std.scad>
right_triangle([80,30], center=true)
show_anchors(std=false);
Function/Module: trapezoid()
Synopsis: Creates a trapezoid with parallel top and bottom sides. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
Usage: As Module
- trapezoid(h, w1, w2, [shift=], [rounding=], [chamfer=], [flip=], ...) [ATTACHMENTS];
- trapezoid(h, w1, ang=, [rounding=], [chamfer=], [flip=], ...) [ATTACHMENTS];
- trapezoid(h, w2=, ang=, [rounding=], [chamfer=], [flip=], ...) [ATTACHMENTS];
- trapezoid(w1=, w2=, ang=, [rounding=], [chamfer=], [flip=], ...) [ATTACHMENTS];
Usage: As Function
- path = trapezoid(...);
Description:
When called as a function, returns a 2D path for a trapezoid with parallel front and back (top and bottom) sides. When called as a module, creates a 2D trapezoid. You can specify the trapezoid by giving its height and the lengths of its two bases. Alternatively, you can omit one of those parameters and specify the lower angle(s). The shift parameter, which cannot be combined with ang, shifts the back (top) of the trapezoid to the right.
Arguments:
By Position | What it does |
---|---|
h |
The Y axis height of the trapezoid. |
w1 |
The X axis width of the front end of the trapezoid. |
w2 |
The X axis width of the back end of the trapezoid. |
By Name | What it does |
---|---|
ang |
Specify the bottom angle(s) of the trapezoid. Can give a scalar for an isosceles trapezoid or a list of two angles, the left angle and right angle. You must omit one of h , w1 , or w2 to allow the freedom to control the angles. |
shift |
Scalar value to shift the back of the trapezoid along the X axis by. Cannot be combined with ang. Default: 0 |
rounding |
The rounding radius for the corners. If given as a list of four numbers, gives individual radii for each corner, in the order [X+Y+,X-Y+,X-Y-,X+Y-]. Default: 0 (no rounding) |
chamfer |
The Length of the chamfer faces at the corners. If given as a list of four numbers, gives individual chamfers for each corner, in the order [X+Y+,X-Y+,X-Y-,X+Y-]. Default: 0 (no chamfer) |
flip |
If true, negative roundings and chamfers will point forward and back instead of left and right. Default: false . |
atype |
The type of anchoring to use with anchor= . Valid opptions are "box" and "perim". This lets you choose between putting anchors on the rounded or chamfered perimeter, or on the square bounding box of the shape. Default: "box" |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Anchor Types:
Anchor Type | What it is |
---|---|
box | Anchor is with respect to the rectangular bounding box of the shape. |
perim | Anchors are placed along the rounded or chamfered perimeter of the shape. |
Example 1:
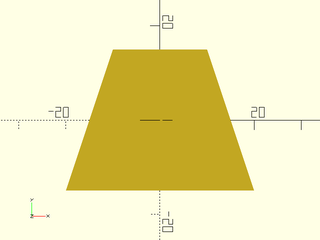
include <BOSL2/std.scad>
trapezoid(h=30, w1=40, w2=20);
Example 2:
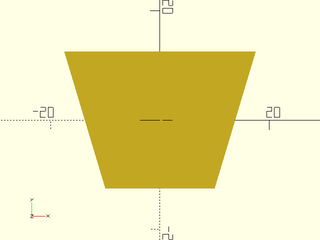
include <BOSL2/std.scad>
trapezoid(h=25, w1=20, w2=35);
Example 3:
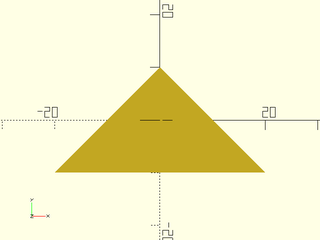
include <BOSL2/std.scad>
trapezoid(h=20, w1=40, w2=0);
Example 4:
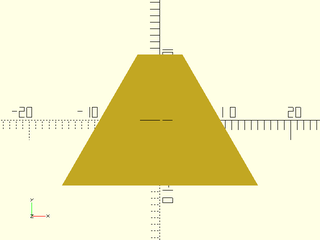
include <BOSL2/std.scad>
trapezoid(h=20, w1=30, ang=60);
Example 5:
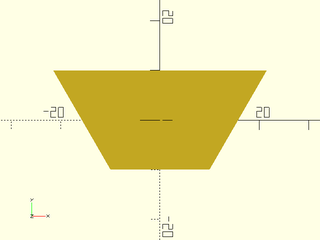
include <BOSL2/std.scad>
trapezoid(h=20, w1=20, ang=120);
Example 6:
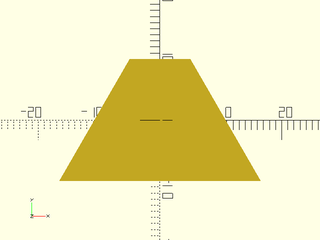
include <BOSL2/std.scad>
trapezoid(h=20, w2=10, ang=60);
Example 7:
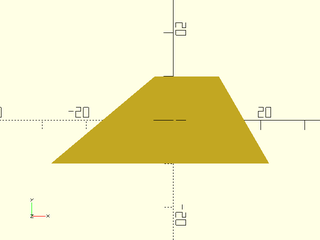
include <BOSL2/std.scad>
trapezoid(h=20, w1=50, ang=[40,60]);
Example 8:
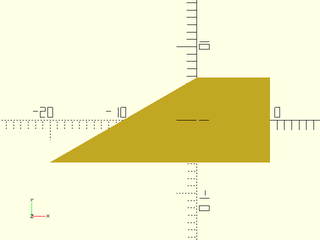
include <BOSL2/std.scad>
trapezoid(w1=30, w2=10, ang=[30,90]);
Example 9: Chamfered Trapezoid
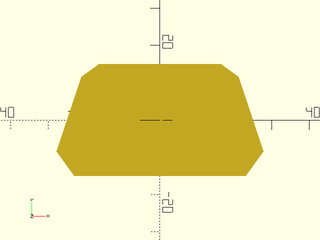
include <BOSL2/std.scad>
trapezoid(h=30, w1=60, w2=40, chamfer=5);
Example 10: Negative Chamfered Trapezoid
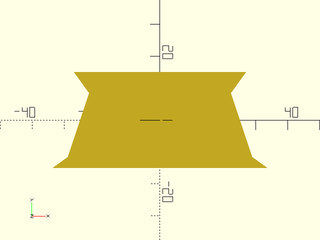
include <BOSL2/std.scad>
trapezoid(h=30, w1=60, w2=40, chamfer=-5);
Example 11: Flipped Negative Chamfered Trapezoid
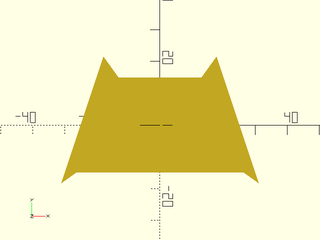
include <BOSL2/std.scad>
trapezoid(h=30, w1=60, w2=40, chamfer=-5, flip=true);
Example 12: Rounded Trapezoid
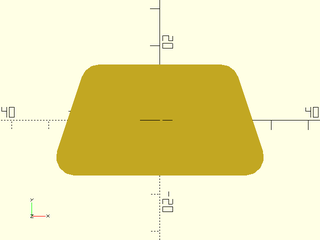
include <BOSL2/std.scad>
trapezoid(h=30, w1=60, w2=40, rounding=5);
Example 13: Negative Rounded Trapezoid
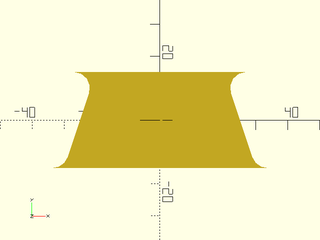
include <BOSL2/std.scad>
trapezoid(h=30, w1=60, w2=40, rounding=-5);
Example 14: Flipped Negative Rounded Trapezoid
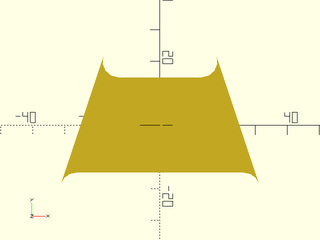
include <BOSL2/std.scad>
trapezoid(h=30, w1=60, w2=40, rounding=-5, flip=true);
Example 15: Mixed Chamfering and Rounding
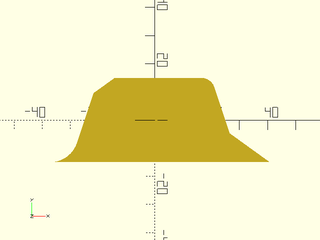
include <BOSL2/std.scad>
trapezoid(h=30, w1=60, w2=40, rounding=[5,0,-10,0],chamfer=[0,8,0,-15],$fa=1,$fs=1);
Example 16: default anchors for roundings
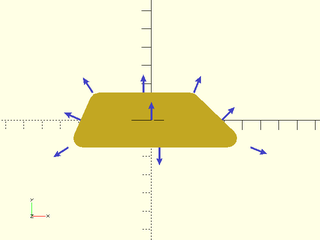
include <BOSL2/std.scad>
trapezoid(h=30, w1=100, ang=[66,44],rounding=5) show_anchors();
Example 17: default anchors for negative roundings are still at the trapezoid corners
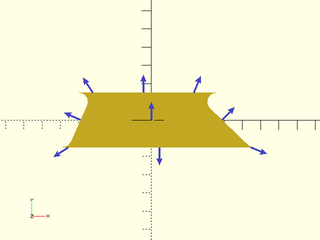
include <BOSL2/std.scad>
trapezoid(h=30, w1=100, ang=[66,44],rounding=-5) show_anchors();
Example 18: "perim" anchors are at the tips of negative roundings
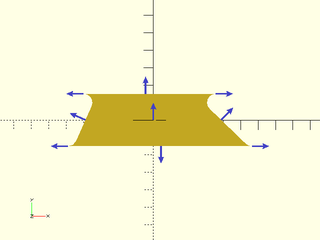
include <BOSL2/std.scad>
trapezoid(h=30, w1=100, ang=[66,44],rounding=-5, atype="perim") show_anchors();
Example 19: They point the other direction if you flip them
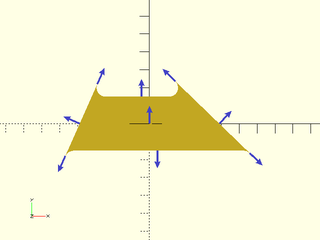
include <BOSL2/std.scad>
trapezoid(h=30, w1=100, ang=[66,44],rounding=-5, atype="perim",flip=true) show_anchors();
Example 20: Called as Function
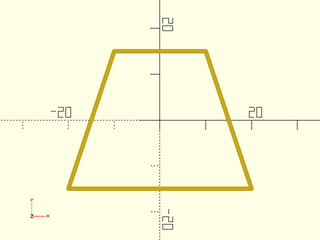
include <BOSL2/std.scad>
stroke(closed=true, trapezoid(h=30, w1=40, w2=20));
Function/Module: star()
Synopsis: Creates a star-shaped polygon or returns a star-shaped region. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: circle(), ellipse(), regular_ngon()
Usage: As Module
- star(n, r/or, ir, [realign=], [align_tip=], [align_pit=], ...) [ATTACHMENTS];
- star(n, r/or, step=, ...) [ATTACHMENTS];
Usage: As Function
- path = star(n, r/or, ir, [realign=], [align_tip=], [align_pit=], ...);
- path = star(n, r/or, step=, ...);
Description:
When called as a function, returns the path needed to create a star polygon with N points. When called as a module, creates a star polygon with N points.
Arguments:
By Position | What it does |
---|---|
n |
The number of stellate tips on the star. |
r / or |
The radius to the tips of the star. |
ir |
The radius to the inner corners of the star. |
By Name | What it does |
---|---|
d / od |
The diameter to the tips of the star. |
id |
The diameter to the inner corners of the star. |
step |
Calculates the radius of the inner star corners by virtually drawing a straight line step tips around the star. 2 <= step < n/2 |
realign |
If false, vertex 0 will lie on the X+ axis. If true then the midpoint of the last edge will lie on the X+ axis, and vertex 0 will be below the X axis. Default: false |
align_tip |
If given as a 2D vector, rotates the whole shape so that the first star tip points in that direction. This occurs before spin. |
align_pit |
If given as a 2D vector, rotates the whole shape so that the first inner corner is pointed towards that direction. This occurs before spin. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
atype |
Choose "hull" or "intersect" anchor methods. Default: "hull" |
Named Anchors:
Anchor Name | Position |
---|---|
"tip0" ... "tip4" | Each tip has an anchor, pointing outwards. |
"pit0" ... "pit4" | The inside corner between each tip has an anchor, pointing outwards. |
"midpt0" ... "midpt4" | The center-point between each pair of tips has an anchor, pointing outwards. |
Example 1:
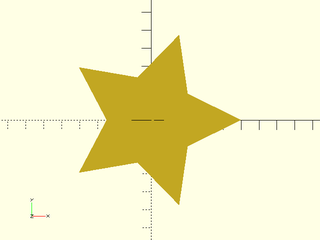
include <BOSL2/std.scad>
star(n=5, r=50, ir=25);
Example 2:
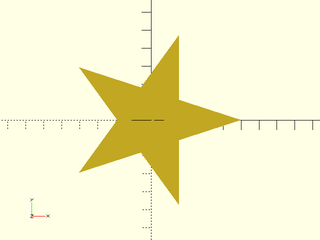
include <BOSL2/std.scad>
star(n=5, r=50, step=2);
Example 3:
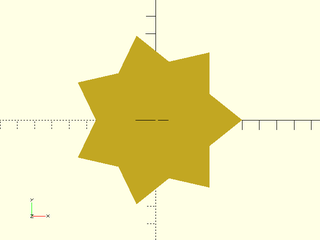
include <BOSL2/std.scad>
star(n=7, r=50, step=2);
Example 4:
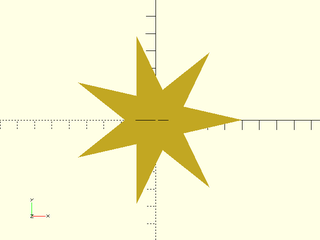
include <BOSL2/std.scad>
star(n=7, r=50, step=3);
Example 5: Realigned
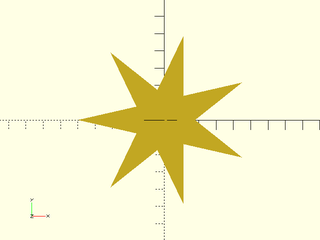
include <BOSL2/std.scad>
star(n=7, r=50, step=3, realign=true);
Example 6: Alignment by Tip
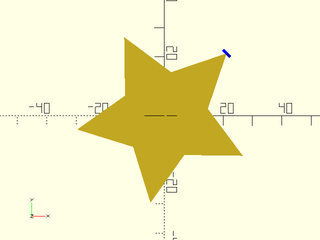
include <BOSL2/std.scad>
star(n=5, ir=15, or=30, align_tip=BACK+RIGHT)
attach("tip0", FWD) color("blue")
stroke([[0,0],[0,7]], endcap2="arrow2");
Example 7: Alignment by Pit
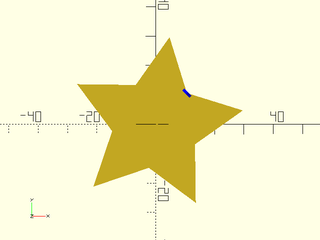
include <BOSL2/std.scad>
star(n=5, ir=15, or=30, align_pit=BACK+RIGHT)
attach("pit0", FWD) color("blue")
stroke([[0,0],[0,7]], endcap2="arrow2");
Example 8: Called as Function
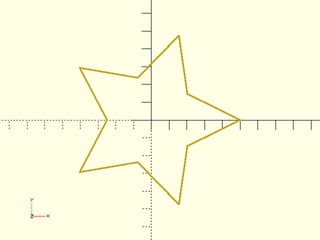
include <BOSL2/std.scad>
stroke(closed=true, star(n=5, r=50, ir=25));
Module: jittered_poly()
Synopsis: Creates a polygon with extra points for smoother twisted extrusions. [Geom]
Topics: Extrusions
See Also: subdivide_path()
Usage:
- jittered_poly(path, [dist]);
Description:
Creates a 2D polygon shape from the given path in such a way that any extra
collinear points are not stripped out in the way that polygon()
normally does.
This is useful for refining the mesh of a linear_extrude()
with twist.
Arguments:
By Position | What it does |
---|---|
path |
The path to add jitter to. |
dist |
The amount to jitter points by. Default: 1/512 (0.00195) |
Example 1:
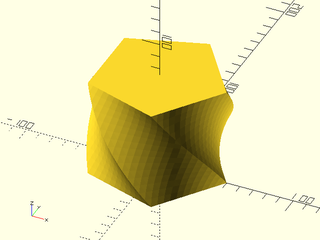
include <BOSL2/std.scad>
d = 100; h = 75; quadsize = 5;
path = pentagon(d=d);
spath = subdivide_path(path, maxlen=quadsize, closed=true);
linear_extrude(height=h, twist=72, slices=h/quadsize)
jittered_poly(spath);
Section: Curved 2D Shapes
Function/Module: teardrop2d()
Synopsis: Creates a 2D teardrop shape. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: teardrop(), onion(), keyhole()
Usage: As Module
- teardrop2d(r/d=, [ang], [cap_h]) [ATTACHMENTS];
Usage: As Function
- path = teardrop2d(r|d=, [ang], [cap_h]);
Description:
When called as a module, makes a 2D teardrop shape. Useful for extruding into 3D printable holes as it limits overhang to 45 degrees. Uses "intersect" style anchoring.
The cap_h parameter truncates the top of the teardrop. If cap_h is taller than the untruncated form then
the result will be the full, untruncated shape. The segments of the bottom section of the teardrop are
calculated to be the same as a circle or cylinder when rotated 90 degrees. (Note that this agreement is poor when $fn=6
or $fn=7
.
If $fn
is a multiple of four then the teardrop will reach its extremes on all four axes. The circum option
produces a teardrop that circumscribes the circle; in this case set realign=true
to get a teardrop that meets its internal extremes
on the axes.
When called as a function, returns a 2D path to for a teardrop shape.
Arguments:
By Position | What it does |
---|---|
r |
radius of circular part of teardrop. (Default: 1) |
ang |
angle of hat walls from the Y axis (half the angle of the peak). (Default: 45 degrees) |
cap_h |
if given, height above center where the shape will be truncated. |
By Name | What it does |
---|---|
d |
diameter of circular portion of bottom. (Use instead of r) |
circum |
if true, create a circumscribing teardrop. Default: false |
realign |
if true, change whether bottom of teardrop is a point or a flat. Default: false |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Example 1: Typical Shape
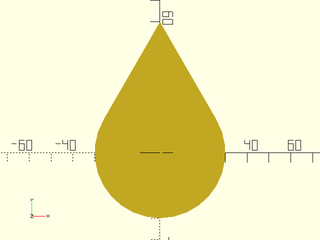
include <BOSL2/std.scad>
teardrop2d(r=30, ang=30);
Example 2: Crop Cap
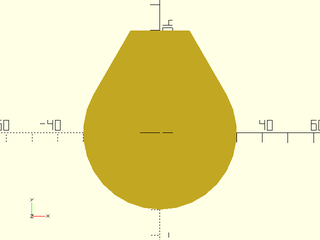
include <BOSL2/std.scad>
teardrop2d(r=30, ang=30, cap_h=40);
Example 3: Close Crop
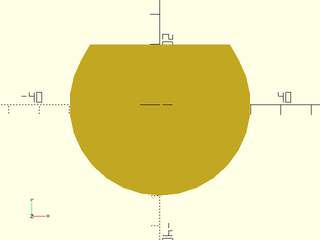
include <BOSL2/std.scad>
teardrop2d(r=30, ang=30, cap_h=20);
Function/Module: egg()
Synopsis: Creates an egg-shaped 2d object. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: circle(), ellipse(), glued_circles(), keyhole()
Usage: As Module
- egg(length, r1|d1=, r2|d2=, R|D=) [ATTACHMENTS];
Usage: As Function
- path = egg(length, r1|d1=, r2|d2=, R|D=);
Description:
When called as a module, constructs an egg-shaped object by connecting two circles with convex arcs that are tangent to the circles.
You specify the length of the egg, the radii of the two circles, and the desired arc radius.
Note that because the side radius, R, is often much larger than the end radii, you may get better
results using $fs
and $fa
to control the number of semgments rather than using $fn
.
This shape may be useful for creating a cam.
When called as a function, returns a 2D path for an egg-shaped object.
Arguments:
By Position | What it does |
---|---|
length |
length of the egg |
r1 |
radius of the left-hand circle |
r2 |
radius of the right-hand circle |
R |
radius of the joining arcs |
By Name | What it does |
---|---|
d1 |
diameter of the left-hand circle |
d2 |
diameter of the right-hand circle |
D |
diameter of the joining arcs |
Named Anchors:
Anchor Name | Position |
---|---|
"left" | center of the left circle |
"right" | center of the right circle |
Example 1: This first example shows how the egg is constructed from two circles and two joining arcs.
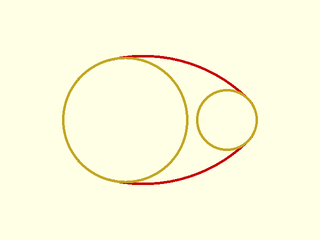
include <BOSL2/std.scad>
$fn=100;
color("red") stroke(egg(78,25,12, 60),closed=true);
stroke([left(14,circle(25)),
right(27,circle(12))]);
Example 2: Varying length between circles
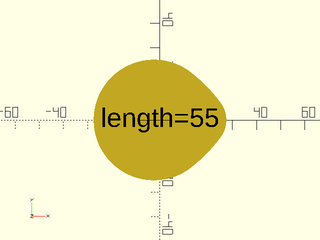
include <BOSL2/std.scad>
r1 = 25; r2 = 12; R = 65;
length = floor(lookup($t, [[0,55], [0.5,90], [1,55]]));
egg(length,r1,r2,R,$fn=180);
color("black") text(str("length=",length), size=8, halign="center", valign="center");
Example 3: Varying tangent arc radius R
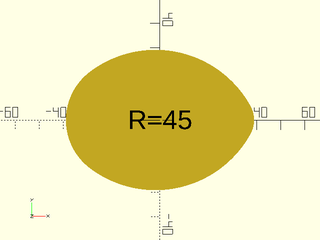
include <BOSL2/std.scad>
length = 78; r1 = 25; r2 = 12;
R = floor(lookup($t, [[0,45], [0.5,150], [1,45]]));
egg(length,r1,r2,R,$fn=180);
color("black") text(str("R=",R), size=8, halign="center", valign="center");
Example 4: Varying circle radius r2
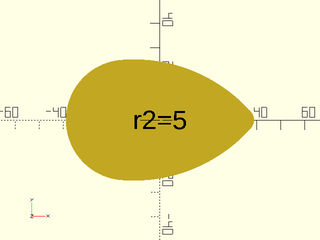
include <BOSL2/std.scad>
length = 78; r1 = 25; R = 65;
r2 = floor(lookup($t, [[0,5], [0.5,30], [1,5]]));
egg(length,r1,r2,R,$fn=180);
color("black") text(str("r2=",r2), size=8, halign="center", valign="center");
Function/Module: ring()
Synopsis: Draws a 2D ring or partial ring or returns a region or path [Geom] [Region] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Regions, Attachable
Usage: ring or partial ring from radii/diameters
- region=ring(n, r1=|d1=, r2=|d2=, [full=], [angle=], [start=]);
Usage: ring or partial ring from radius and ring width
- region=ring(n, ring_width, r=|d=, [full=], [angle=], [start=]);
Usage: ring or partial ring passing through three points
- region=ring(n, [ring_width], [r=,d=], points=[P0,P1,P2], [full=]);
Usage: ring or partial ring from tangent point on segment [P0,P1]
to the tangent point on segment [P1,P2]
.
- region=ring(n, [ring_width], corner=[P0,P1,P2], [r=,d=], [r1|d1=], [r2=|d2=], [full=]);
Usage: ring or partial ring based on setting a width at the X axis and height above the X axis
- region=ring(n, [ring_width], [r=|d=], width=, thickness=, [full=]);
Usage: as a module
- ring(...) [ATTACHMENTS];
Description:
If called as a function returns a region or path for a ring or part of a ring. If called as a module, creates the corresponding 2D ring or partial ring shape.
The geometry of the ring can be specified using any of the methods supported by arc()
. If full
is true (the default) the ring will be complete and the
returned value a region. If full
is false then the return is a path describing a partial ring. The returned path is always clockwise with the larger radius arc first.
A ring has two radii, the inner and outer. When specifying geometry you must somehow specify one radius, which can be directly with r=
or r1=
or by giving a point list with
or without a center point. You specify the second radius by giving r=
directly, or r2=
if you used r1=
for the first radius, or by giving ring_width
. If ring_width
the second radius will be larger than the first; if ring_width
is negative the second radius will be smaller.
Arguments:
By Position | What it does |
---|---|
n |
Number of vertices to use for the inner and outer portions of the ring |
ring_width |
width of the ring. Can be positive or negative |
By Name | What it does |
---|---|
r1 / d1 |
inner radius or diameter of the ring |
r2 / d2 |
outer radius or diameter of the ring |
r / d |
second radius or diameter of ring when r1 or d1 are not given |
full |
if true create a full ring, if false create a partial ring. Default: true unless angle is given |
cp |
Centerpoint of ring. |
points |
Points on the ring boundary. |
corner |
A path of two segments to fit the ring tangent to. |
long |
if given with cp and points takes the long arc instead of the default short arc. Default: false |
cw |
if given with cp and 2 points takes the arc in the clockwise direction. Default: false |
ccw |
if given with cp and 2 points takes the arc in the counter-clockwise direction. Default: false |
width |
If given with thickness , ring is defined based on an arc with ends on X axis. |
thickness |
If given with width , ring is defined based on an arc with ends on X axis, and this height above the X axis. |
start |
Start angle of ring. Default: 0 |
angle |
If scalar, the end angle in degrees relative to start parameter. If a vector specifies start and end angles of ring. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. (Module only) Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. (Module only) Default: 0 |
Example 1:
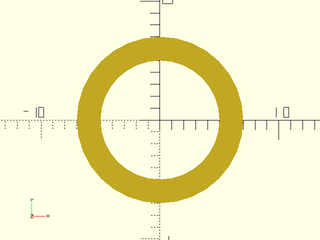
include <BOSL2/std.scad>
ring(r1=5,r2=7, n=32);
Example 2:
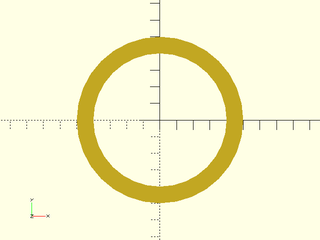
include <BOSL2/std.scad>
ring(r=5,ring_width=-1, n=32);
Example 3:
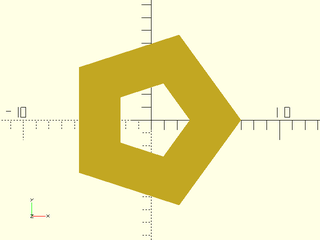
include <BOSL2/std.scad>
ring(r=7, n=5, ring_width=-4);
Example 4:
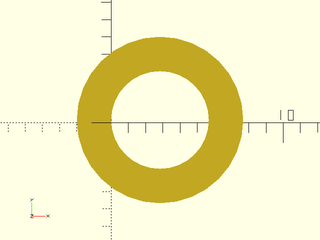
include <BOSL2/std.scad>
ring(points=[[0,0],[3,3],[5,2]], ring_width=2, n=32);
Example 5:
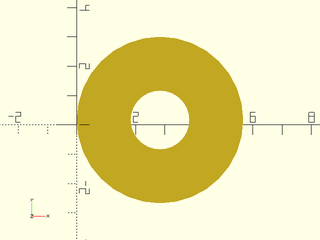
include <BOSL2/std.scad>
ring(points=[[0,0],[3,3],[5,2]], r=1, n=32);
Example 6:
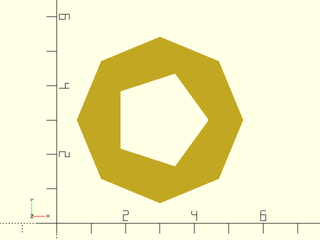
include <BOSL2/std.scad>
ring(cp=[3,3], points=[[4,4],[1,3]], ring_width=1);
Example 7:
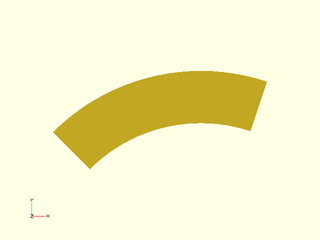
include <BOSL2/std.scad>
ring(corner=[[0,0],[4,4],[7,3]], r2=2, r1=1.5,n=22,full=false);
Example 8:
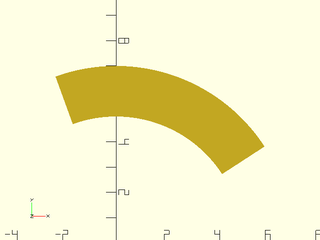
include <BOSL2/std.scad>
ring(r1=5,r2=7, angle=[33,110], n=32);
Example 9:
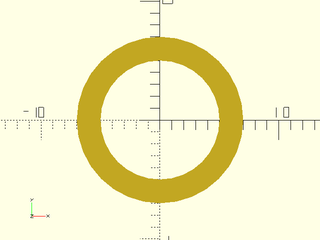
include <BOSL2/std.scad>
ring(r1=5,r2=7, angle=[0,360], n=32); // full circle
Example 10:
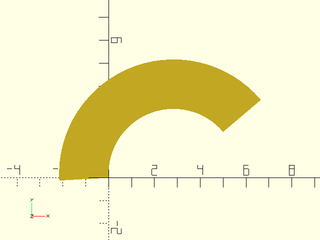
include <BOSL2/std.scad>
ring(r=5, points=[[0,0],[3,3],[5,2]], full=false, n=32);
Example 11:
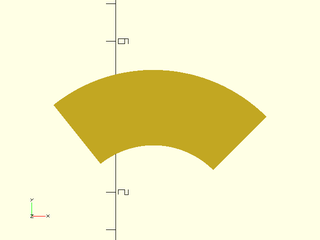
include <BOSL2/std.scad>
ring(32,-2, cp=[1,1], points=[[4,4],[-3,6]], full=false);
Example 12:
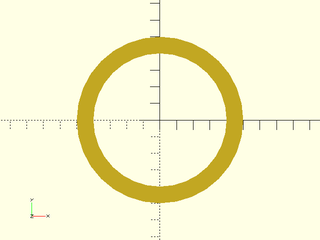
include <BOSL2/std.scad>
ring(r=5,ring_width=-1, n=32);
Example 13:
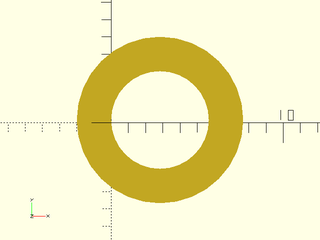
include <BOSL2/std.scad>
ring(points=[[0,0],[3,3],[5,2]], ring_width=2, n=32);
Example 14:
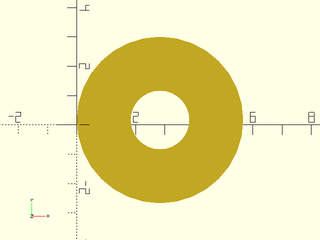
include <BOSL2/std.scad>
ring(points=[[0,0],[3,3],[5,2]], r=1, n=32);
Example 15:
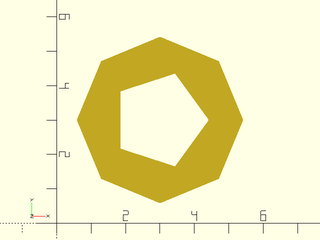
include <BOSL2/std.scad>
ring(cp=[3,3], points=[[4,4],[1,3]], ring_width=1);
Example 16: Using corner, the outer radius is the one tangent to the corner
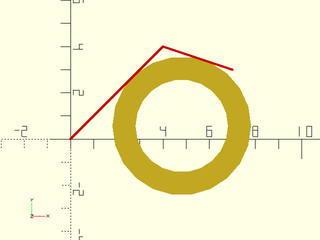
include <BOSL2/std.scad>
corner = [[0,0],[4,4],[7,3]];
ring(corner=corner, r2=3, r1=2,n=22);
stroke(corner, width=.1,color="red");
Example 17: For inner radius tangent to a corner, specify r=
and ring_width
.
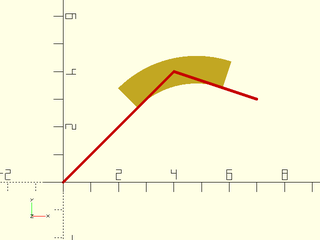
include <BOSL2/std.scad>
corner = [[0,0],[4,4],[7,3]];
ring(corner=corner, r=3, ring_width=1,n=22,full=false);
stroke(corner, width=.1,color="red");
Example 18:
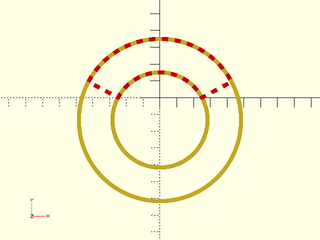
include <BOSL2/std.scad>
$fn=128;
region = ring(width=5,thickness=1.5,ring_width=2);
path = ring(width=5,thickness=1.5,ring_width=2,full=false);
stroke(region,width=.25);
color("red") dashed_stroke(path,dashpat=[1.5,1.5],closed=true,width=.25);
Function/Module: glued_circles()
Synopsis: Creates a shape of two circles joined by a curved waist. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: circle(), ellipse(), egg(), keyhole()
Usage: As Module
- glued_circles(r/d=, [spread], [tangent], ...) [ATTACHMENTS];
Usage: As Function
- path = glued_circles(r/d=, [spread], [tangent], ...);
Description:
When called as a function, returns a 2D path forming a shape of two circles joined by curved waist. When called as a module, creates a 2D shape of two circles joined by curved waist. Uses "hull" style anchoring.
Arguments:
By Position | What it does |
---|---|
r |
The radius of the end circles. |
spread |
The distance between the centers of the end circles. Default: 10 |
tangent |
The angle in degrees of the tangent point for the joining arcs, measured away from the Y axis. Default: 30 |
By Name | What it does |
---|---|
d |
The diameter of the end circles. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Example 1:
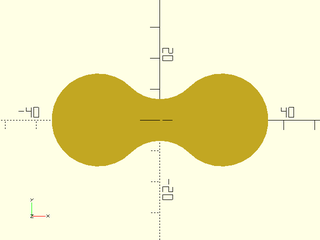
include <BOSL2/std.scad>
glued_circles(r=15, spread=40, tangent=45);
Example 2:
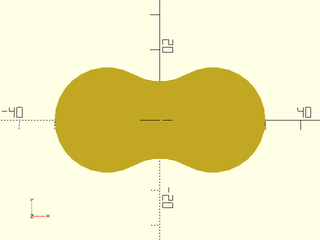
include <BOSL2/std.scad>
glued_circles(d=30, spread=30, tangent=30);
Example 3:
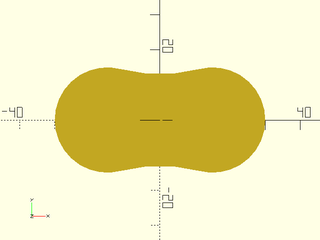
include <BOSL2/std.scad>
glued_circles(d=30, spread=30, tangent=15);
Example 4:
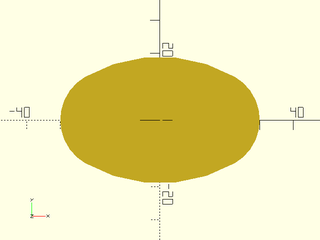
include <BOSL2/std.scad>
glued_circles(d=30, spread=30, tangent=-30);
Example 5: Called as Function
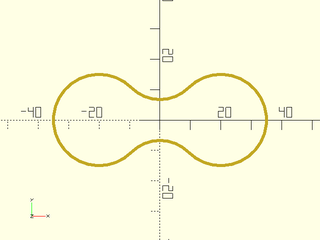
include <BOSL2/std.scad>
stroke(closed=true, glued_circles(r=15, spread=40, tangent=45));
Function/Module: squircle()
Synopsis: Creates a shape between a circle and a square, centered on the origin. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: circle(), square(), supershape()
Usage: As Module
- squircle(size, [squareness], [style]) [ATTACHMENTS];
Usage: As Function
- path = squircle(size, [squareness], [style]);
Description:
A squircle is a shape intermediate between a square/rectangle and a circle/ellipse. Squircles are sometimes used to make dinner plates (more area for the same radius as a circle), keyboard buttons, and smartphone icons. Old CRT television screens also resembled elongated squircles.
Multiple definitions exist for the squircle. We support two versions: the superellipse supershape()
Example 3, also known as the Lamé upper squircle), and the Fernandez-Guasti squircle. They are visually almost indistinguishable, with the superellipse having slightly rounder "corners" than FG at the same corner radius. These two squircles have different, unintuitive methods for controlling how square or circular the shape is. A squareness
parameter determines the shape, specifying the corner position linearly, with 0 being on the circle and 1 being the square. Vertices are positioned to be more dense near the corners to preserve smoothness at low values of $fn
'.
For the "superellipse" style, the special case where the superellipse exponent is 4 results in a squircle at the geometric mean between radial points on the circle and square, corresponding to squareness=0.456786.
When called as a module, creates a 2D squircle with the desired squareness. When called as a function, returns a 2D path for a squircle.
Arguments:
By Position | What it does |
---|---|
size |
Same as the size parameter in square() , can be a single number or a vector [xsize,ysize] . |
squareness |
Value between 0 and 1. Controls the shape, setting the location of a squircle "corner" at the specified interpolated position between a circle and a square. When squareness=0 the shape is a circle, and when squareness=1 the shape is a square. Default: 0.5 |
By Name | What it does |
---|---|
style |
method for generating a squircle, "fg" for Fernández-Guasti and "superellipse" for superellipse. Default: "fg" |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
atype |
anchor type, "box" for bounding box corners and sides, "perim" for the squircle corners. Default: "box" |
$fn |
Number of points. The special variables $fs and $fa are ignored. If set, $fn must be 12 or greater, and is rounded to the nearest multiple of 4. Points are generated so they are more dense around sharper curves. Default if not set: 48 |
Example 1:
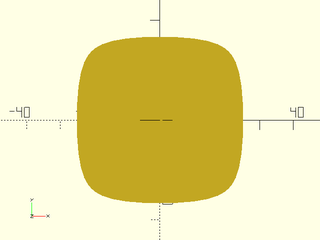
include <BOSL2/std.scad>
squircle(size=50, squareness=0.4);
Example 2:
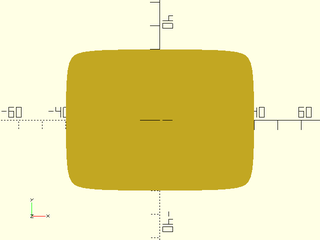
include <BOSL2/std.scad>
squircle([80,60], 0.7, $fn=64);
Example 3: Ten increments of squareness parameter for a superellipse squircle
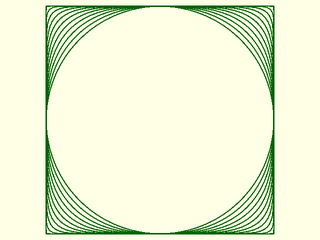
include <BOSL2/std.scad>
color("green") for(sq=[0:0.1:1])
stroke(squircle(100, sq, style="superellipse", $fn=96), closed=true, width=0.5);
Example 4: Standard vector anchors are based on the bounding box
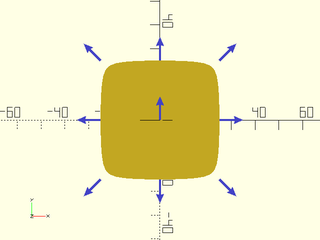
include <BOSL2/std.scad>
squircle(50, 0.6) show_anchors();
Example 5: Perimeter anchors, anchoring at bottom left and spinning 20°
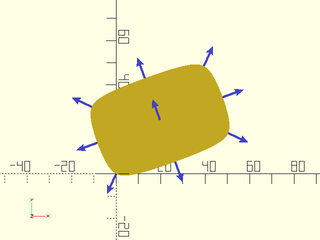
include <BOSL2/std.scad>
squircle([60,40], 0.5, anchor=(BOTTOM+LEFT), atype="perim", spin=20)
show_anchors();
Function/Module: keyhole()
Synopsis: Creates a 2D keyhole shape. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: circle(), ellipse(), egg(), glued_circles()
Usage: As Module
- keyhole(l/length=, r1/d1=, r2/d2=, [shoulder_r=], ...) [ATTACHMENTS];
Usage: As Function
- path = keyhole(l/length=, r1/d1=, r2/d2=, [shoulder_r=], ...);
Description:
When called as a function, returns a 2D path forming a shape of two differently sized circles joined by a straight slot, making what looks like a keyhole. When called as a module, creates a 2D shape of two differently sized circles joined by a straight slot, making what looks like a keyhole. Uses "hull" style anchoring.
Arguments:
By Position | What it does |
---|---|
l |
The distance between the centers of the two circles. Default: 15 |
r1 |
The radius of the back circle, centered on [0,0] . Default: 2.5 |
r2 |
The radius of the forward circle, centered on [0,-length] . Default: 5 |
By Name | What it does |
---|---|
shoulder_r |
The radius of the rounding of the shoulder between the larger circle, and the slot that leads to the smaller circle. Default: 0 |
d1 |
The diameter of the back circle, centered on [0,0] . |
d2 |
The diameter of the forward circle, centered on [0,-l] . |
length |
An alternate name for the l= argument. |
anchor |
Translate so anchor point is at origin (0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Example 1:
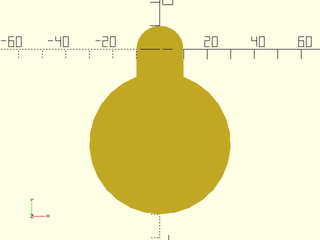
include <BOSL2/std.scad>
keyhole(40, 10, 30);
Example 2:
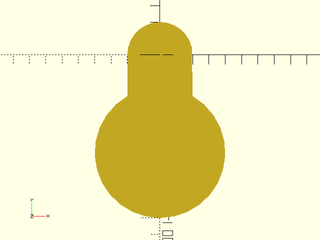
include <BOSL2/std.scad>
keyhole(l=60, r1=20, r2=40);
Example 3: Making the forward circle larger than the back circle
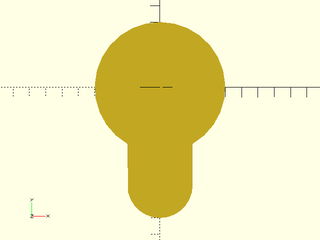
include <BOSL2/std.scad>
keyhole(l=60, r1=40, r2=20);
Example 4: Centering on the larger hole:
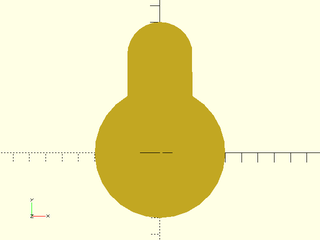
include <BOSL2/std.scad>
keyhole(l=60, r1=40, r2=20, spin=180);
Example 5: Rounding the shoulders
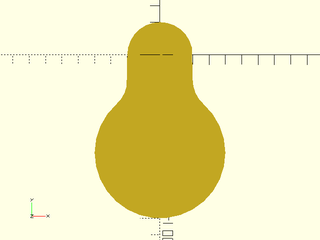
include <BOSL2/std.scad>
keyhole(l=60, r1=20, r2=40, shoulder_r=20);
Example 6: Called as Function
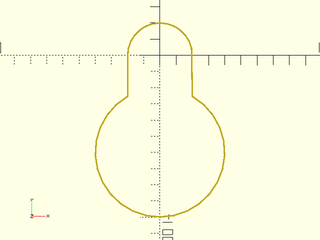
include <BOSL2/std.scad>
stroke(closed=true, keyhole(l=60, r1=20, r2=40));
Function/Module: supershape()
Synopsis: Creates a 2D Superformula shape. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
Usage: As Module
- supershape([step],[n=], [m1=], [m2=], [n1=], [n2=], [n3=], [a=], [b=], [r=/d=]) [ATTACHMENTS];
Usage: As Function
- path = supershape([step], [n=], [m1=], [m2=], [n1=], [n2=], [n3=], [a=], [b=], [r=/d=]);
Description:
When called as a function, returns a 2D path for the outline of the Superformula shape. When called as a module, creates a 2D Superformula shape. Note that the "hull" type anchoring (the default) is more intuitive for concave star-like shapes, but the anchor points do not necesarily lie on the line of the anchor vector, which can be confusing, especially for simpler, ellipse-like shapes. Note that the default step angle of 0.5 is very fine and can be slow, but due to the complex curves of the supershape, many points are often required to give a good result.
Arguments:
By Position | What it does |
---|---|
step |
The angle step size for sampling the superformula shape. Smaller steps are slower but more accurate. Default: 0.5 |
By Name | What it does |
---|---|
n |
Produce n points as output. Alternative to step. Not to be confused with shape parameters n1 and n2. |
m1 |
The m1 argument for the superformula. Default: 4. |
m2 |
The m2 argument for the superformula. Default: m1. |
n1 |
The n1 argument for the superformula. Default: 1. |
n2 |
The n2 argument for the superformula. Default: n1. |
n3 |
The n3 argument for the superformula. Default: n2. |
a |
The a argument for the superformula. Default: 1. |
b |
The b argument for the superformula. Default: a. |
r |
Radius of the shape. Scale shape to fit in a circle of radius r. |
d |
Diameter of the shape. Scale shape to fit in a circle of diameter d. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
atype |
Select "hull" or "intersect" style anchoring. Default: "hull". |
Example 1:
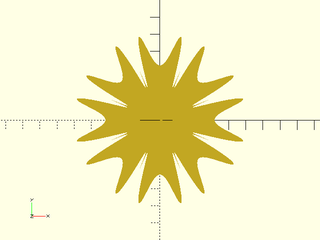
include <BOSL2/std.scad>
supershape(step=0.5,m1=16,m2=16,n1=0.5,n2=0.5,n3=16,r=50);
Example 2: Called as Function
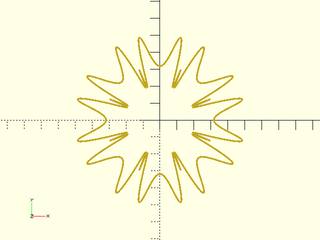
include <BOSL2/std.scad>
stroke(closed=true, supershape(step=0.5,m1=16,m2=16,n1=0.5,n2=0.5,n3=16,d=100));
Example 3:
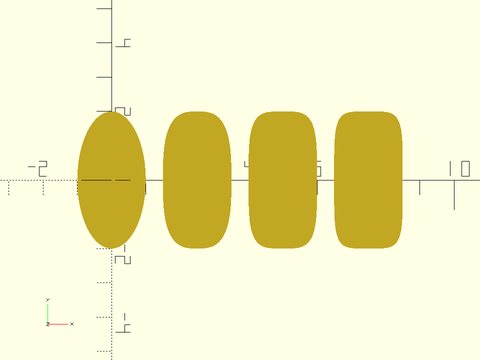
include <BOSL2/std.scad>
for(n=[2:5]) right(2.5*(n-2)) supershape(m1=4,m2=4,n1=n,a=1,b=2); // Superellipses
Example 4:
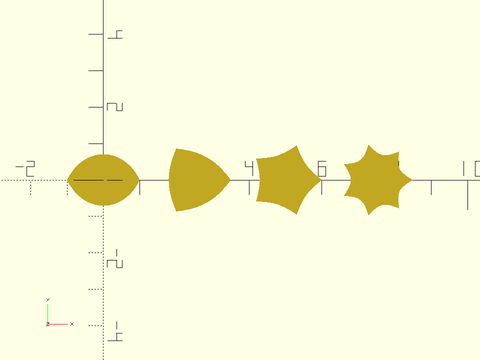
include <BOSL2/std.scad>
m=[2,3,5,7]; for(i=[0:3]) right(2.5*i) supershape(.5,m1=m[i],n1=1);
Example 5:
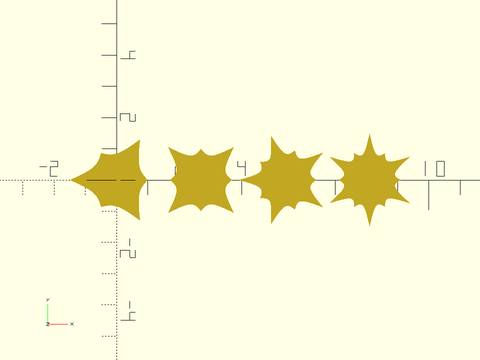
include <BOSL2/std.scad>
m=[6,8,10,12]; for(i=[0:3]) right(2.7*i) supershape(.5,m1=m[i],n1=1,b=1.5); // m should be even
Example 6:
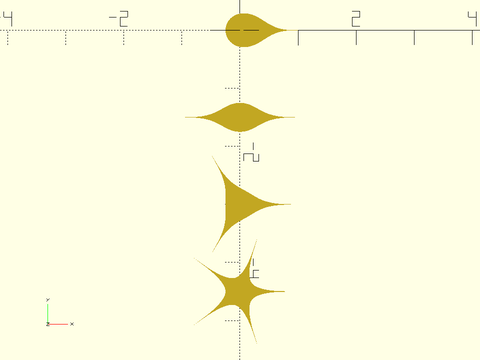
include <BOSL2/std.scad>
m=[1,2,3,5]; for(i=[0:3]) fwd(1.5*i) supershape(m1=m[i],n1=0.4);
Example 7:
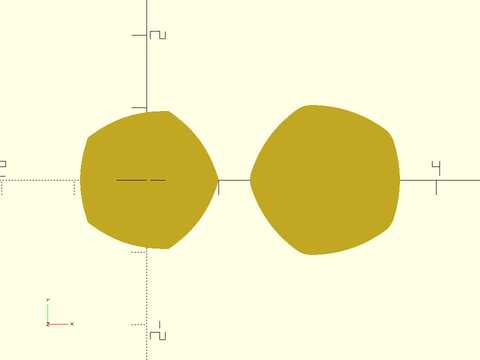
include <BOSL2/std.scad>
supershape(m1=5, n1=4, n2=1); right(2.5) supershape(m1=5, n1=40, n2=10);
Example 8:
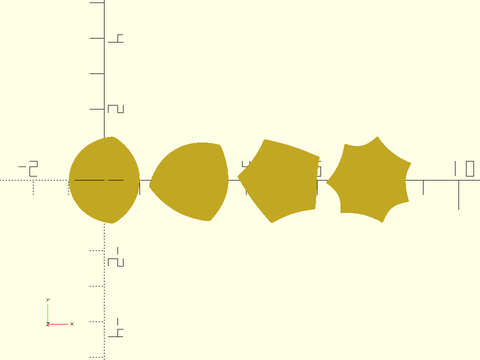
include <BOSL2/std.scad>
m=[2,3,5,7]; for(i=[0:3]) right(2.5*i) supershape(m1=m[i], n1=60, n2=55, n3=30);
Example 9:
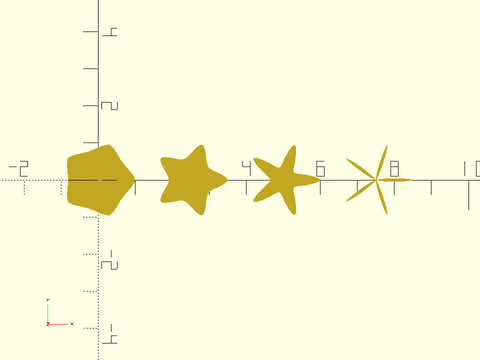
include <BOSL2/std.scad>
n=[0.5,0.2,0.1,0.02]; for(i=[0:3]) right(2.5*i) supershape(m1=5,n1=n[i], n2=1.7);
Example 10:
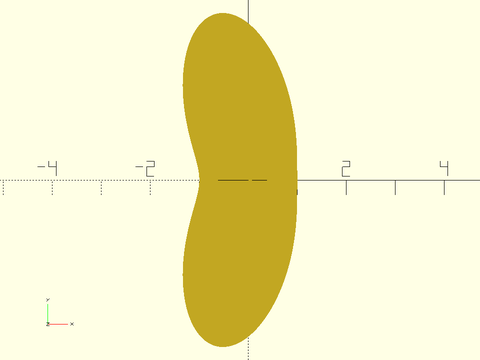
include <BOSL2/std.scad>
supershape(m1=2, n1=1, n2=4, n3=8);
Example 11:
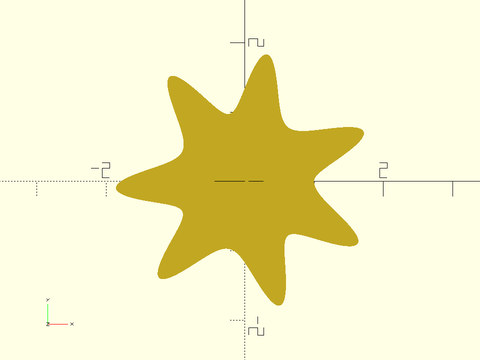
include <BOSL2/std.scad>
supershape(m1=7, n1=2, n2=8, n3=4);
Example 12:
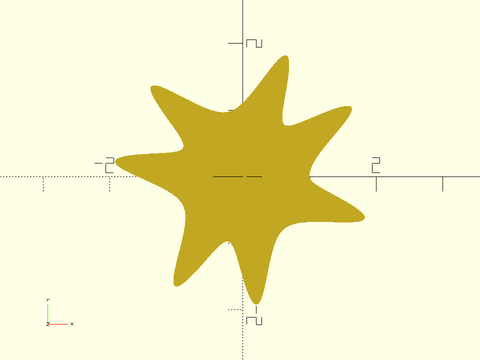
include <BOSL2/std.scad>
supershape(m1=7, n1=3, n2=4, n3=17);
Example 13:
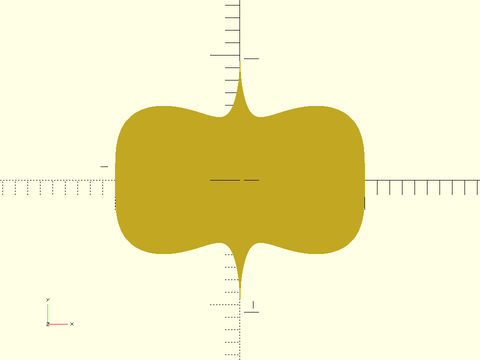
include <BOSL2/std.scad>
supershape(m1=4, n1=1/2, n2=1/2, n3=4);
Example 14:
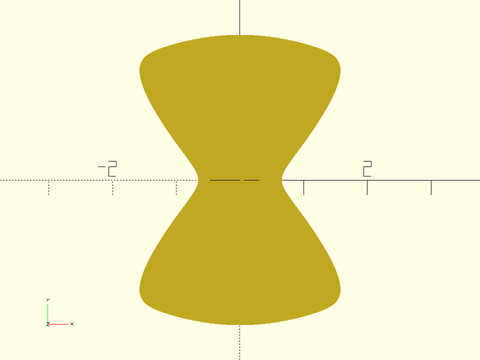
include <BOSL2/std.scad>
supershape(m1=4, n1=4.0,n2=16, n3=1.5, a=0.9, b=9);
Example 15:
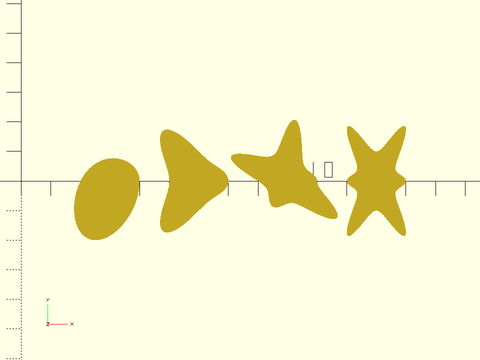
include <BOSL2/std.scad>
for(i=[1:4]) right(3*i) supershape(m1=i, m2=3*i, n1=2);
Example 16:
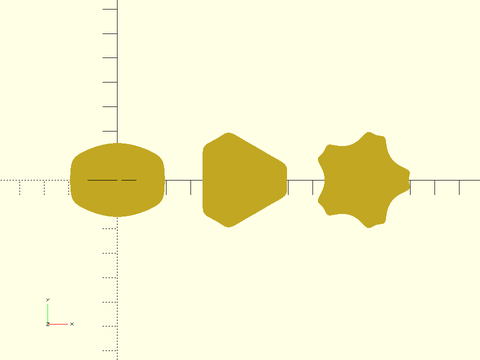
include <BOSL2/std.scad>
m=[4,6,10]; for(i=[0:2]) right(i*5) supershape(m1=m[i], n1=12, n2=8, n3=5, a=2.7);
Example 17:
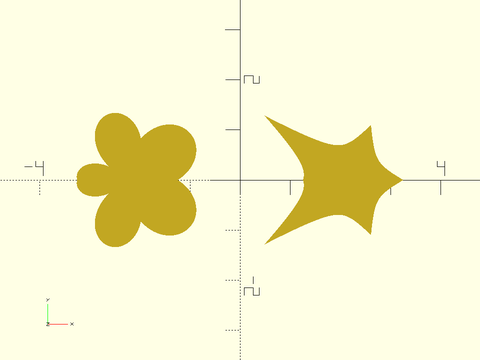
include <BOSL2/std.scad>
for(i=[-1.5:3:1.5]) right(i*1.5) supershape(m1=2,m2=10,n1=i,n2=1);
Example 18:
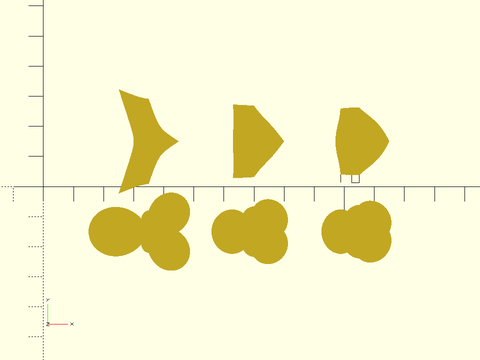
include <BOSL2/std.scad>
for(i=[1:3],j=[-1,1]) translate([3.5*i,1.5*j])supershape(m1=4,m2=6,n1=i*j,n2=1);
Example 19:
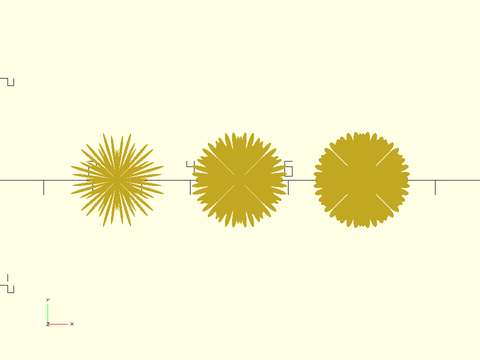
include <BOSL2/std.scad>
for(i=[1:3]) right(2.5*i)supershape(step=.5,m1=88, m2=64, n1=-i*i,n2=1,r=1);
Example 20:
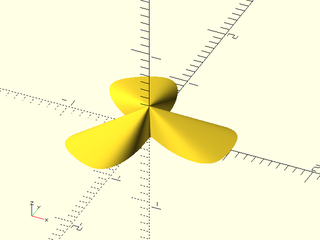
include <BOSL2/std.scad>
linear_extrude(height=0.3, scale=0) supershape(step=1, m1=6, n1=0.4, n2=0, n3=6);
Example 21:
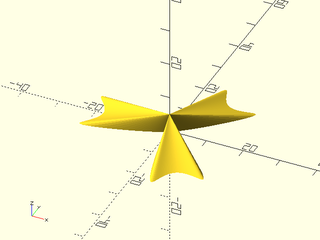
include <BOSL2/std.scad>
linear_extrude(height=5, scale=0) supershape(step=1, b=3, m1=6, n1=3.8, n2=16, n3=10);
Function/Module: reuleaux_polygon()
Synopsis: Creates a constant-width shape that is not circular. [Geom] [Path]
Topics: Shapes (2D), Paths (2D), Path Generators, Attachable
See Also: regular_ngon(), pentagon(), hexagon(), octagon()
Usage: As Module
- reuleaux_polygon(n, r|d=, ...) [ATTACHMENTS];
Usage: As Function
- path = reuleaux_polygon(n, r|d=, ...);
Description:
When called as a module, creates a 2D Reuleaux Polygon; a constant width shape that is not circular. Uses "intersect" type anchoring. When called as a function, returns a 2D path for a Reulaux Polygon.
Arguments:
By Position | What it does |
---|---|
n |
Number of "sides" to the Reuleaux Polygon. Must be an odd positive number. Default: 3 |
r |
Radius of the shape. Scale shape to fit in a circle of radius r. |
By Name | What it does |
---|---|
d |
Diameter of the shape. Scale shape to fit in a circle of diameter d. |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
Named Anchors:
Anchor Name | Position |
---|---|
"tip0", "tip1", etc. | Each tip has an anchor, pointing outwards. |
Example 1:
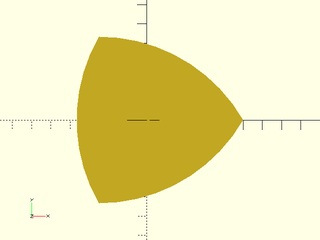
include <BOSL2/std.scad>
reuleaux_polygon(n=3, r=50);
Example 2:
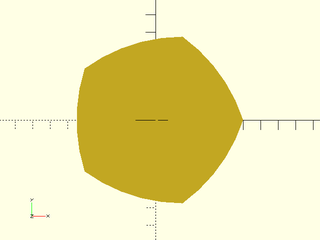
include <BOSL2/std.scad>
reuleaux_polygon(n=5, d=100);
Example 3: Standard vector anchors are based on extents
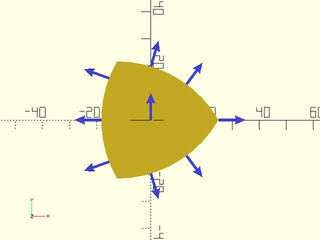
include <BOSL2/std.scad>
reuleaux_polygon(n=3, d=50) show_anchors(custom=false);
Example 4: Named anchors exist for the tips
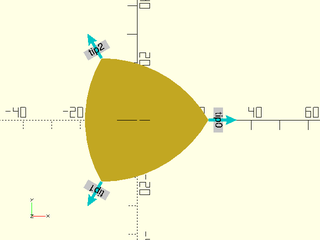
include <BOSL2/std.scad>
reuleaux_polygon(n=3, d=50) show_anchors(std=false);
Section: Text
Module: text()
Synopsis: Creates an attachable block of text. [Geom]
Topics: Attachments, Text
See Also: text3d(), attachable()
Usage:
- text(text, [size], [font], ...);
Description:
Creates a 3D text block that can be attached to other attachable objects. You cannot attach children to text.
Historically fonts were specified by their "body size", the height of the metal body on which the glyphs were cast. This means the size was an upper bound on the size of the font glyphs, not a direct measurement of their size. In digital typesetting, the metal body is replaced by an invisible box, the em square, whose side length is defined to be the font's size. The glyphs can be contained in that square, or they can extend beyond it, depending on the choices made by the font designer. As a result, the meaning of font size varies between fonts: two fonts at the "same" size can differ significantly in the actual size of their characters. Typographers customarily specify the size in the units of "points". A point is 1/72 inch. In OpenSCAD, you specify the size in OpenSCAD units (often treated as millimeters for 3d printing), so if you want points you will need to perform a suitable unit conversion. In addition, the OpenSCAD font system has a bug: if you specify size=s you will instead get a font whose size is s/0.72. For many fonts this means the size of capital letters will be approximately equal to s, because it is common for fonts to use about 70% of their height for the ascenders in the font. To get the customary font size, you should multiply your desired size by 0.72.
To find the fonts that you have available in your OpenSCAD installation, go to the Help menu and select "Font List".
Arguments:
By Position | What it does |
---|---|
text |
Text to create. |
size |
The font will be created at this size divided by 0.72. Default: 10 |
font |
Font to use. Default: "Liberation Sans" (standard OpenSCAD default) |
By Name | What it does |
---|---|
halign |
If given, specifies the horizontal alignment of the text. "left" , "center" , or "right" . Overrides anchor= . |
valign |
If given, specifies the vertical alignment of the text. "top" , "center" , "baseline" or "bottom" . Overrides anchor= . |
spacing |
The relative spacing multiplier between characters. Default: 1.0 |
direction |
The text direction. "ltr" for left to right. "rtl" for right to left. "ttb" for top to bottom. "btt" for bottom to top. Default: "ltr" |
language |
The language the text is in. Default: "en" |
script |
The script the text is in. Default: "latin" |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: "baseline" |
spin |
Rotate this many degrees around the Z axis. See spin. Default: 0 |
Named Anchors:
Anchor Name | Position |
---|---|
"baseline" | Anchors at the baseline of the text, at the start of the string. |
str("baseline",VECTOR) | Anchors at the baseline of the text, modified by the X and Z components of the appended vector. |
Example 1:
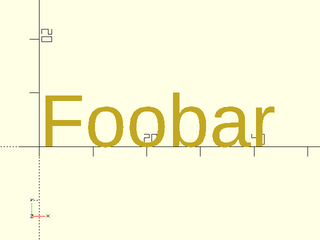
include <BOSL2/std.scad>
text("Foobar", size=10);
Example 2:
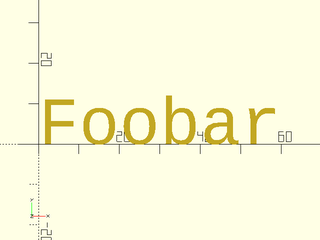
include <BOSL2/std.scad>
text("Foobar", size=12, font="Liberation Mono");
Example 3:
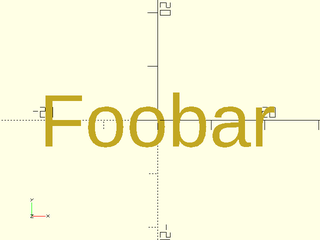
include <BOSL2/std.scad>
text("Foobar", anchor=CENTER);
Example 4:
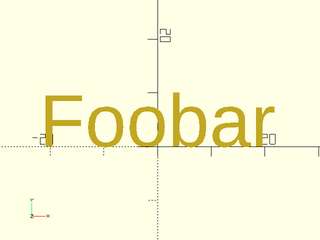
include <BOSL2/std.scad>
text("Foobar", anchor=str("baseline",CENTER));
Example 5: Using line_copies() distributor
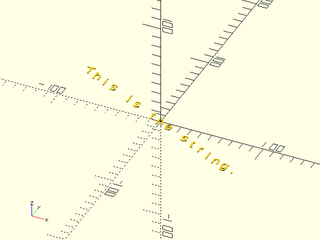
include <BOSL2/std.scad>
txt = "This is the string.";
line_copies(spacing=[10,-5],n=len(txt))
text(txt[$idx], size=10, anchor=CENTER);
Example 6: Using arc_copies() distributor
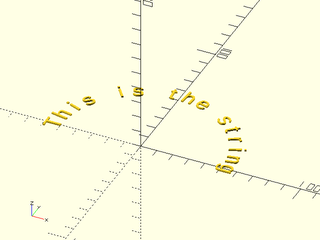
include <BOSL2/std.scad>
txt = "This is the string";
arc_copies(r=50, n=len(txt), sa=0, ea=180)
text(select(txt,-1-$idx), size=10, anchor=str("baseline",CENTER), spin=-90);
Section: Rounding 2D shapes
Module: round2d()
Synopsis: Rounds the corners of 2d objects. [Geom]
Topics: Rounding
See Also: shell2d(), round3d(), minkowski_difference()
Usage:
- round2d(r) [ATTACHMENTS];
- round2d(or=) [ATTACHMENTS];
- round2d(ir=) [ATTACHMENTS];
- round2d(or=, ir=) [ATTACHMENTS];
Description:
Rounds arbitrary 2D objects. Giving r
rounds all concave and convex corners. Giving just ir
rounds just concave corners. Giving just or
rounds convex corners. Giving both ir
and or
can let you round to different radii for concave and convex corners. The 2D object must not have
any parts narrower than twice the or
radius. Such parts will disappear.
Arguments:
By Position | What it does |
---|---|
r |
Radius to round all concave and convex corners to. |
By Name | What it does |
---|---|
or |
Radius to round only outside (convex) corners to. Use instead of r . |
ir |
Radius to round only inside (concave) corners to. Use instead of r . |
Example 1:
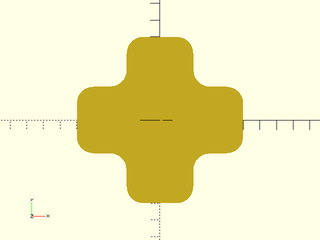
include <BOSL2/std.scad>
round2d(r=10) {square([40,100], center=true); square([100,40], center=true);}
Example 2:
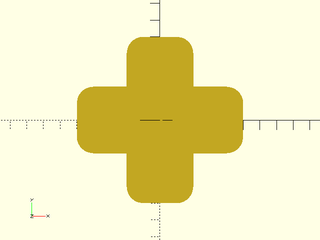
include <BOSL2/std.scad>
round2d(or=10) {square([40,100], center=true); square([100,40], center=true);}
Example 3:
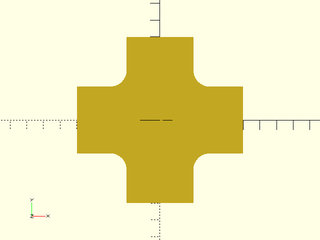
include <BOSL2/std.scad>
round2d(ir=10) {square([40,100], center=true); square([100,40], center=true);}
Example 4:
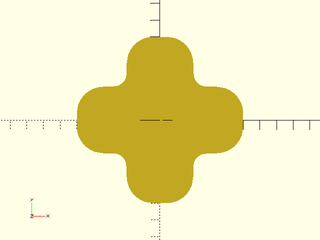
include <BOSL2/std.scad>
round2d(or=16,ir=8) {square([40,100], center=true); square([100,40], center=true);}
Module: shell2d()
Synopsis: Creates a shell from 2D children. [Geom]
Topics: Shell
See Also: round2d(), round3d(), minkowski_difference()
Usage:
- shell2d(thickness, [or], [ir])
Description:
Creates a hollow shell from 2D children, with optional rounding.
Arguments:
By Position | What it does |
---|---|
thickness |
Thickness of the shell. Positive to expand outward, negative to shrink inward, or a two-element list to do both. |
or |
Radius to round corners on the outside of the shell. If given a list of 2 radii, [CONVEX,CONCAVE], specifies the radii for convex and concave corners separately. Default: 0 (no outside rounding) |
ir |
Radius to round corners on the inside of the shell. If given a list of 2 radii, [CONVEX,CONCAVE], specifies the radii for convex and concave corners separately. Default: 0 (no inside rounding) |
Example 1:
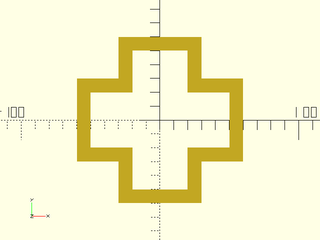
include <BOSL2/std.scad>
shell2d(10) {square([40,100], center=true); square([100,40], center=true);}
Example 2:
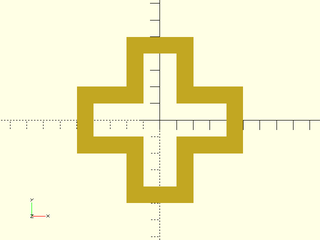
include <BOSL2/std.scad>
shell2d(-10) {square([40,100], center=true); square([100,40], center=true);}
Example 3:
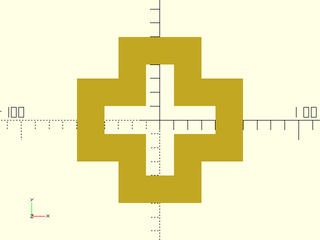
include <BOSL2/std.scad>
shell2d([-10,10]) {square([40,100], center=true); square([100,40], center=true);}
Example 4:
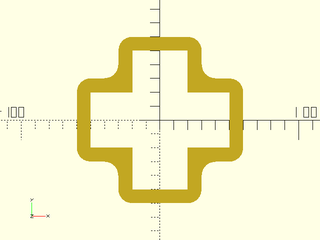
include <BOSL2/std.scad>
shell2d(10,or=10) {square([40,100], center=true); square([100,40], center=true);}
Example 5:
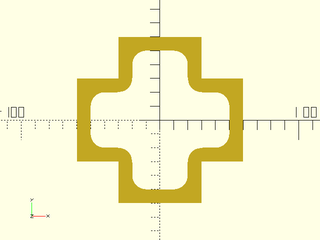
include <BOSL2/std.scad>
shell2d(10,ir=10) {square([40,100], center=true); square([100,40], center=true);}
Example 6:
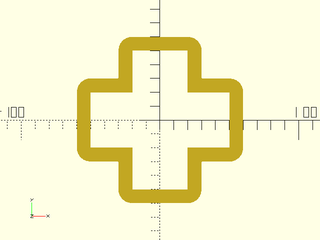
include <BOSL2/std.scad>
shell2d(10,or=[10,0]) {square([40,100], center=true); square([100,40], center=true);}
Example 7:
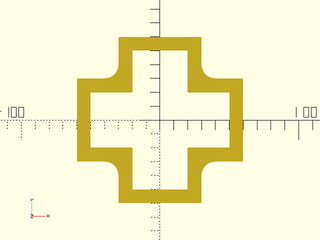
include <BOSL2/std.scad>
shell2d(10,or=[0,10]) {square([40,100], center=true); square([100,40], center=true);}
Example 8:
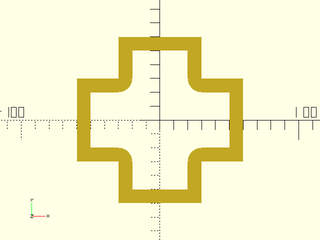
include <BOSL2/std.scad>
shell2d(10,ir=[10,0]) {square([40,100], center=true); square([100,40], center=true);}
Example 9:
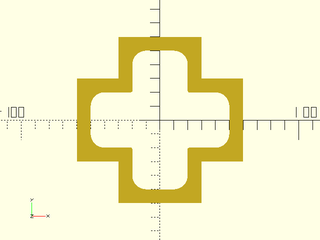
include <BOSL2/std.scad>
shell2d(10,ir=[0,10]) {square([40,100], center=true); square([100,40], center=true);}
Example 10:
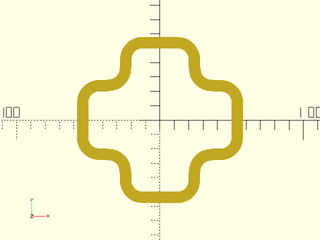
include <BOSL2/std.scad>
shell2d(8,or=[16,8],ir=[16,8]) {square([40,100], center=true); square([100,40], center=true);}
Indices
Table of Contents
Function Index
Topics Index
Cheat Sheet
Tutorials
List of Files:
Basic Modeling:
- constants.scad STD
- transforms.scad STD
- attachments.scad STD
- shapes2d.scad STD
- shapes3d.scad STD
- drawing.scad STD
- masks2d.scad STD
- masks3d.scad STD
- distributors.scad STD
- color.scad STD
- partitions.scad STD
- miscellaneous.scad STD
Advanced Modeling:
- paths.scad STD
- regions.scad STD
- skin.scad STD
- vnf.scad STD
- beziers.scad
- nurbs.scad
- rounding.scad
- turtle3d.scad
Math:
- math.scad STD
- linalg.scad STD
- vectors.scad STD
- coords.scad STD
- geometry.scad STD
- trigonometry.scad STD
Data Management:
- version.scad STD
- comparisons.scad STD
- lists.scad STD
- utility.scad STD
- strings.scad STD
- structs.scad STD
- fnliterals.scad
Threaded Parts:
Parts:
- ball_bearings.scad
- cubetruss.scad
- gears.scad
- hinges.scad
- joiners.scad
- linear_bearings.scad
- modular_hose.scad
- nema_steppers.scad
- polyhedra.scad
- sliders.scad
- tripod_mounts.scad
- walls.scad
- wiring.scad
Footnotes:
STD = Included in std.scad