This file contains Unicode characters that might be confused with other characters. If you think that this is intentional, you can safely ignore this warning. Use the Escape button to reveal them.
LibFile: screw_drive.scad
Masks for Phillips, Torx and square (Robertson) driver holes.
To use, add the following lines to the beginning of your file:
include <BOSL2/std.scad>
include <BOSL2/screw_drive.scad>
File Contents
-
phillips_mask()
– Creates a mask for a Philips screw drive. [Geom]phillips_depth()
– Returns the depth a phillips recess needs to be for a given diameter.phillips_diam()
– Returns the diameter of a phillips recess of a given depth.
-
hex_drive_mask()
– Creates a mask for a hex drive recess. [Geom]
-
torx_mask()
– Creates a mask for a torx drive recess. [Geom]torx_mask2d()
– Creates the 2D cross section for a torx drive recess. [Geom]torx_info()
– Returns the dimensions of a torx drive.torx_diam()
– Returns the diameter of a torx drive.torx_depth()
– Returns the typical depth of a torx drive recess.
-
Section: Robertson/Square Drives
robertson_mask()
– Creates a mask for a Robertson/Square drive recess. [Geom]
Section: Phillips Drive
Module: phillips_mask()
Synopsis: Creates a mask for a Philips screw drive. [Geom]
See Also: hex_drive_mask(), phillips_depth(), phillips_diam(), torx_mask(), robertson_mask()
Usage:
- phillips_mask(size) [ATTACHMENTS];
Description:
Creates a mask for creating a Phillips drive recess given the Phillips size. Each mask can be lowered to different depths to create different sizes of recess.
Arguments:
By Position | What it does |
---|---|
size |
The size of the bit as an integer or string. "#0", "#1", "#2", "#3", or "#4" |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
orient |
Vector to rotate top towards, after spin. See orient. Default: UP |
Example 1:
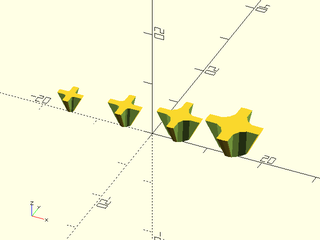
include <BOSL2/std.scad>
include <BOSL2/screw_drive.scad>
xdistribute(10) {
phillips_mask(size="#1");
phillips_mask(size="#2");
phillips_mask(size=3);
phillips_mask(size=4);
}
Function: phillips_depth()
Synopsis: Returns the depth a phillips recess needs to be for a given diameter.
See Also: phillips_mask(), hex_drive_mask(), phillips_diam(), torx_mask()
Usage:
- depth = phillips_depth(size, d);
Description:
Returns the depth of the Phillips recess required to produce the specified diameter, or undef if not possible.
Arguments:
By Position | What it does |
---|---|
size |
size as a number or text string like "#2" |
d |
desired diameter |
Function: phillips_diam()
Synopsis: Returns the diameter of a phillips recess of a given depth.
See Also: phillips_mask(), hex_drive_mask(), phillips_depth(), torx_mask()
Usage:
- diam = phillips_diam(size, depth);
Description:
Returns the diameter at the top of the Phillips recess when constructed at the specified depth, or undef if that depth is not valid.
Arguments:
By Position | What it does |
---|---|
size |
size as number or text string like "#2" |
depth |
depth of recess to find the diameter of |
Section: Hex drive
Module: hex_drive_mask()
Synopsis: Creates a mask for a hex drive recess. [Geom]
See Also: phillips_mask(), torx_mask(), phillips_depth(), phillips_diam(), robertson_mask()
Usage:
- hex_drive_mask(size, length, [anchor], [spin], [orient], [$slop]) [ATTACHMENTS];
Description:
Creates a mask for hex drive. Note that the hex recess specs requires
a slightly oversized recess. You can use $slop to increase the size by
2 * $slop
if necessary.
Section: Torx Drive
Module: torx_mask()
Synopsis: Creates a mask for a torx drive recess. [Geom]
See Also: phillips_mask(), hex_drive_mask(), phillips_depth(), phillips_diam(), robertson_mask()
Usage:
- torx_mask(size, l, [center]) [ATTACHMENTS];
Description:
Creates a torx bit tip. The anchors are located on the circumscribing cylinder. See torx_info()
for allowed sizes.
Arguments:
By Position | What it does |
---|---|
size |
Torx size. |
l |
Length of bit. |
center |
If true, centers mask vertically. |
By Name | What it does |
---|---|
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: CENTER |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
orient |
Vector to rotate top towards, after spin. See orient. Default: UP |
Example 1:
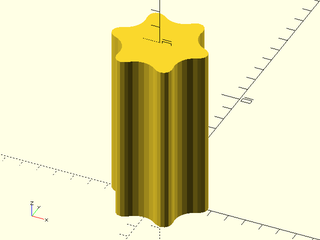
include <BOSL2/std.scad>
include <BOSL2/screw_drive.scad>
torx_mask(size=30, l=10, $fa=1, $fs=1);
Module: torx_mask2d()
Synopsis: Creates the 2D cross section for a torx drive recess. [Geom]
See Also: phillips_mask(), hex_drive_mask(), torx_mask(), phillips_depth(), phillips_diam(), torx_info(), robertson_mask()
Usage:
- torx_mask2d(size);
Description:
Creates a torx bit 2D profile. The anchors are located on the circumscribing circle. See torx_info()
for allowed sizes.
Arguments:
By Position | What it does |
---|---|
size |
Torx size. |
Example 1:
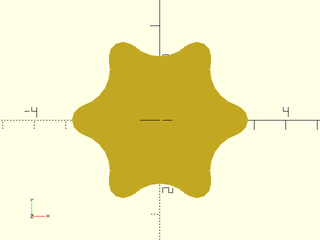
include <BOSL2/std.scad>
include <BOSL2/screw_drive.scad>
torx_mask2d(size=30, $fa=1, $fs=1);
Function: torx_info()
Synopsis: Returns the dimensions of a torx drive.
See Also: phillips_mask(), hex_drive_mask(), torx_mask(), phillips_depth(), phillips_diam()
Usage:
- info = torx_info(size);
Description:
Get the typical dimensional info for a given Torx size. Returns a list containing, in order:
- Outer Diameter
- Inner Diameter
- Drive Hole Depth
- External Tip Rounding Radius
- Inner Rounding Radius
Arguments:
By Position | What it does |
---|---|
size |
Torx size. |
Function: torx_diam()
Synopsis: Returns the diameter of a torx drive.
See Also: phillips_mask(), hex_drive_mask(), torx_mask(), phillips_depth(), phillips_diam(), torx_info()
Usage:
- diam = torx_diam(size);
Description:
Get the typical outer diameter of Torx profile.
Arguments:
By Position | What it does |
---|---|
size |
Torx size. |
Function: torx_depth()
Synopsis: Returns the typical depth of a torx drive recess.
See Also: phillips_mask(), hex_drive_mask(), torx_mask(), phillips_depth(), phillips_diam(), torx_info()
Usage:
- depth = torx_depth(size);
Description:
Gets typical drive hole depth.
Arguments:
By Position | What it does |
---|---|
size |
Torx size. |
Section: Robertson/Square Drives
Module: robertson_mask()
Synopsis: Creates a mask for a Robertson/Square drive recess. [Geom]
See Also: phillips_mask(), hex_drive_mask(), torx_mask(), phillips_depth(), phillips_diam(), torx_info()
Usage:
- robertson_mask(size, [extra], [ang], [$slop=]);
Description:
Creates a mask for creating a Robertson/Square drive recess given the drive size as an integer.
The width of the recess will be oversized by 2 * $slop
. Note that this model is based
on an incomplete spec. https://www.aspenfasteners.com/content/pdf/square_drive_specification.pdf
We determined the angle by doing print tests on a Prusa MK3S with $slop set to 0.05.
Arguments:
By Position | What it does |
---|---|
size |
The size of the square drive, as an integer from 0 to 4. |
extra |
Extra length of drive mask to create. |
ang |
taper angle of each face. Default: 2.5 |
By Name | What it does |
---|---|
$slop |
enlarge recess by this twice amount. Default: 0 |
anchor |
Translate so anchor point is at origin (0,0,0). See anchor. Default: TOP |
spin |
Rotate this many degrees around the Z axis after anchor. See spin. Default: 0 |
orient |
Vector to rotate top towards, after spin. See orient. Default: UP |
Side Effects:
- Sets tag to "remove" if no tag is set.
Example 1:
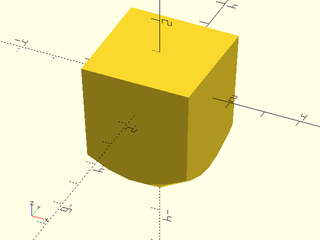
include <BOSL2/std.scad>
include <BOSL2/screw_drive.scad>
robertson_mask(size=2);
Example 2:
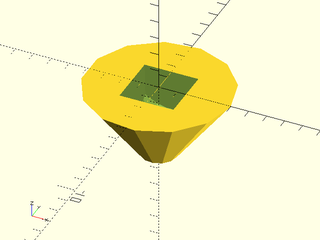
include <BOSL2/std.scad>
include <BOSL2/screw_drive.scad>
difference() {
cyl(d1=2, d2=8, h=4, anchor=TOP);
robertson_mask(size=2);
}
Indices
Table of Contents
Function Index
Topics Index
Cheat Sheet
Tutorials
List of Files:
Basic Modeling:
- constants.scad STD
- transforms.scad STD
- attachments.scad STD
- shapes2d.scad STD
- shapes3d.scad STD
- drawing.scad STD
- masks2d.scad STD
- masks3d.scad STD
- distributors.scad STD
- color.scad STD
- partitions.scad STD
- miscellaneous.scad STD
Advanced Modeling:
- paths.scad STD
- regions.scad STD
- skin.scad STD
- vnf.scad STD
- beziers.scad
- nurbs.scad
- rounding.scad
- turtle3d.scad
Math:
- math.scad STD
- linalg.scad STD
- vectors.scad STD
- coords.scad STD
- geometry.scad STD
- trigonometry.scad STD
Data Management:
- version.scad STD
- comparisons.scad STD
- lists.scad STD
- utility.scad STD
- strings.scad STD
- structs.scad STD
- fnliterals.scad
Threaded Parts:
Parts:
- ball_bearings.scad
- cubetruss.scad
- gears.scad
- hinges.scad
- joiners.scad
- linear_bearings.scad
- modular_hose.scad
- nema_steppers.scad
- polyhedra.scad
- sliders.scad
- tripod_mounts.scad
- walls.scad
- wiring.scad
Footnotes:
STD = Included in std.scad